Simple DirectMedia Layer 2.0
Since 1998, Simple DirectMedia Layer (SDL) has formed the basis for countless games, multimedia applications, and even virtualization. The small cross-platform library makes it easy for applications to access the graphics card, the audio hardware, the keyboard, and other input devices. Because it is available for many different platforms and operating systems, you can easily port any programs that have been developed with it (see the "Supported Operating Systems" box). Without SDL, Linux users would probably still be waiting for conversions of blockbuster games like Portal (Figures 1 and 2).
For an amazing 12 years, SDL version 1.2 provided the underpinnings of numerous programs. Changes have only come in small doses, with the last revision (1.2.15) appearing in January 2012. SDL 1.2 is thus considered extremely robust, but hardware development has mercilessly left it behind. In the background, SDL developers have already been working for several years in parallel on a revamped, modern variant.
The developer versions initially had a version number of 1.3, but – because of the many internal changes – the developers made the leap to 2.0 in February 2012. Although this new branch had already been used in many software projects, the developers were reluctant to put a "Stable" stamp on it. In August 2013, however, SDL inventor and project manager Sam Lantinga drew the line and officially released the current state of development as version 2.0.0.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
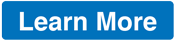
News
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.