Custom solutions for system monitoring and control
Reacting to Logins
From these building blocks, you can create small scripts with easy tools that respond to events on your system. For example, you can quite easily use logins to trigger actions – for example, to avoid discussions with the kids every evening if they have been sitting in front of the computer too long. When the bell tolls, you can then log them off the system automatically whether they are happy with it or not.
The script in Listing 8 relies on users
to discover the users currently logged on to the system and then on grep
to filter the output. This gives you an exit code of
in case of a match and of 1
in case of a miss. Based on the results, and the current time (assuming the hours between 21:00 and 07:00 are off limits), the script then allows user simon to use the system or throws him out without so much as a by your leave (Figure 4).
Listing 8
Automatic Logoff
01 #! /bin/sh 02 while true; do 03 # Discover the time 04 TIMENOW=$(date +%H) 05 # Is Simon logged on? 06 users | grep -q simon 07 # Evaluate exit code 08 A=$? 09 if [ $A -eq 0 ]; then 10 # Simon is logged on 11 if [ $TIMENOW -le 7 ] || [ $TIMENOW -ge 21 ]; then 12 # before 07:00 or after 21:00 hours 13 killall -u simon 14 fi 15 fi 16 sleep 60 17 done
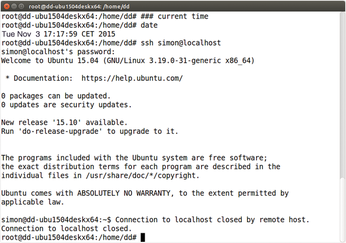
The principle can also be applied to convenience and automation functions. For example, with a slightly modified script (Listing 9) you can launch a web server as soon as a specific user (jefe in this example) logs on. In this case, the script is designed for use with a systemd computer; you might also need to change the name for the web server service (in this example, httpd.service).
Listing 9
Launching a Web Server Conditionally
01 #! /bin/sh 02 while true; do 03 # Is jefe logged on? 04 users | grep -q jefe 05 # Evaluate exit code 06 A=$? 07 if [ $A -eq 0 ]; then 08 # jefe logged on 09 # Check status of web server 10 systemctl status httpd.service 11 # Evaluate exit code 12 B=$? 13 # Start web server if needed 14 if [ $B -gt 0 ]; then 15 systemctl start httpd.service 16 fi 17 else 18 # jefe not logged on 19 # Check status of web server 20 systemctl status httpd.service 21 # Evaluate exit code 22 C=$? 23 if [ $C -eq 0 ]; then 24 # Stop web server if needed 25 systemctl stop httpd.service 26 fi 27 fi 28 sleep 60 29 done
The user does not need to enter any commands or initiate any actions, and you don't even need to make any changes to home directories or user accounts. For example, in ~/.profile
or ~/.bashrc
), all you need is an active session. This approach lets you assign important tasks, such as starting a service or a backup, to a non-privileged user account. Of course, you need to call the shell script automatically when the computer boots. The approach is different, depending on the init system.
As a general rule, you would want to store the executable shell script in the /usr/local/sbin
directory. For older systems, such as Debian 7 with SysVinit, you would typically copy the skeleton
template, which is already in place on most systems, to a new file with an appropriate name below /etc/init.d
(this example uses weblogon
). Next, edit the init script to suit your needs, save the changes, and make the file executable by running chmod +x
. To enable the script for the typical runlevel, enter:
update-rc.d weblogon defaults
If you work on a computer with systemd, you need to create a new unit file named weblogon.service
, as shown in Listing 10, under the /usr/local/lib/systemd/system
directory, which you might have to create, and run the code in Listing 11 to link the instructions with the system and ensure an automatic start at bootup.
Listing 10
weblogon.service
[Unit] Description=Start web server when jefe logs on Documentation=man:users(1) [Service] ExecStart=/usr/local/sbin/webmeldung.sh IgnoreSIGPIPE=false [Install] WantedBy=multi-user.target
Listing 11
Setting Up Autostart at Bootup
$ sudo systemctl enable weblogon.service Created symlink from /etc/systemd/system/multi-user.target. wants/weblogon.service to /usr/local/lib/systemd/system/weblogon.service. $ sudo systemctl start weblogon.service $ sudo systemctl status weblogon.service * weblogon.service - Start web server when jefe logs on Loaded: loaded (/usr/local/lib/systemd/system/weblogon. service; enabled; vendor preset: disabled) Active: active (running) since Tue 2015-10-27 14:16:13 CET; 6s ago [...]
Messages via Email
Optionally, you can also tell your computer to notify you by email in case of important events. To do so, you need a mail transport agent (MTA), such as SSMTP [1] on your computer. The agent forwards email via a regular SMTP server so that the server mail is not discarded automatically as spam by the receiving email service.
Listing 12 shows a simple shell script that notifies you of all users still logged on to the computer after 22:00 hours. To handle this task, the script creates a helper file named mail.txt
, which ssmtp
then uses as input for the email to be sent.
Listing 12
User Notification
01 #!/bin/sh 02 RECEIVERADDRESS=daddy@example.com 03 SENDERADDRESS=kidsroom@home.net 04 while true; do 05 timenow=$(date +%H) 06 if [ $timenow -eq 22 ]; then 07 echo "To: $RECEIVERADDRESS" > mail.txt 08 echo "From: $SENDERADDRESS" >> mail.txt 09 echo "Subject: User query" >> mail.txt 10 echo "" >> mail.txt 11 users >> mail.txt 12 ssmtp $RECEIVERADDRESS < mail.txt 13 sleep 15 14 #sleep 3600 15 fi 16 done
Before starting the script, you first need to set up SSMTP. To do so, use the configuration files ssmtp.conf
(Listing 13) and revaliases
(Listing 14) from the /etc/ssmtp
directory. Depending on which email provider you want to address, you need to enter different configuration details at this point. If you are having difficulty finding the right information, searching the Internet with <Provider> ssmtp will typically help.
Listing 13
ssmtp.conf
root=daddy@example.com mailhub=smtp.example.com:25 hostname=kidsroom UseTLS=Yes UseSTARTTLS=YES AuthUser=<Login for SMTP Server> AuthPass=<Password for SMTP Server> FromLineOverride=NO
Listing 14
revaliases
root:daddy@example.com:smtp.example.com:25
The examples in the configuration files are applicable for a normal FreeMail provider. For test purposes, you can run ssmtp
in the shell script with the -v
option. The program is then far more verbose, allowing you to discover problems with the transmission more quickly and make the necessary changes.
Make sure that only root, or the user in whose context SSMTP runs, can see the SSMTP configuration file. Check the documentation of your Linux distribution for instructions on doing this (e.g., for Arch Linux [2]).
Conclusions
The examples shown here only cover a fraction of the possibilities that shell scripts offer for system monitoring. To create scripts, you do not need advanced programming capabilities; experience with terminal applications and simple constructs such as while
loops and if
statements are typically sufficient.
Infos
- SSMTP: https://packages.qa.debian.org/s/ssmtp.html
- SSMTP security: https://wiki.archlinux.org/index.php/SSMTP#Security
« Previous 1 2
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
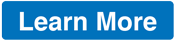
News
-
Gnome 47.1 Released with a Few Fixes
The latest release of the Gnome desktop is all about fixing a few nagging issues and not about bringing new features into the mix.
-
System76 Unveils an Ampere-Powered Thelio Desktop
If you're looking for a new desktop system for developing autonomous driving and software-defined vehicle solutions. System76 has you covered.
-
VirtualBox 7.1.4 Includes Initial Support for Linux kernel 6.12
The latest version of VirtualBox has arrived and it not only adds initial support for kernel 6.12 but another feature that will make using the virtual machine tool much easier.
-
New Slimbook EVO with Raw AMD Ryzen Power
If you're looking for serious power in a 14" ultrabook that is powered by Linux, Slimbook has just the thing for you.
-
The Gnome Foundation Struggling to Stay Afloat
The foundation behind the Gnome desktop environment is having to go through some serious belt-tightening due to continued financial problems.
-
Thousands of Linux Servers Infected with Stealth Malware Since 2021
Perfctl is capable of remaining undetected, which makes it dangerous and hard to mitigate.
-
Halcyon Creates Anti-Ransomware Protection for Linux
As more Linux systems are targeted by ransomware, Halcyon is stepping up its protection.
-
Valve and Arch Linux Announce Collaboration
Valve and Arch have come together for two projects that will have a serious impact on the Linux distribution.
-
Hacker Successfully Runs Linux on a CPU from the Early ‘70s
From the office of "Look what I can do," Dmitry Grinberg was able to get Linux running on a processor that was created in 1971.
-
OSI and LPI Form Strategic Alliance
With a goal of strengthening Linux and open source communities, this new alliance aims to nurture the growth of more highly skilled professionals.