Python graphics libraries for data visualization
Pinpoint Accuracy
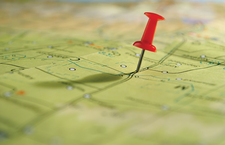
© Lead Image © Sergii Gnatiuk, 123RF.com
Python's powerful Matplotlib, Bokeh, PyQtGraph, and Pandas libraries lend programmers a helping hand when visualizing complex data and their relationships.
Daily life is inundated by data collected, processed, and made available again in edited form. Examples include weather, temperature, precipitation, humidity, air pressure, wind, sales, and load measurements, as well as vehicle and services data.
A first step is to evaluate data in the simplest form as a table that provides an overview of individual values. Beyond this, a suitable graphical visualization helps you to understand the relationships at a glance. Python has excellent libraries to implement this visualization step, including Matplotlib [1], PyQtGraph [2], Bokeh [3], and Pandas [4]. Appropriate libraries for other languages are summarized the "Visualization in Other Languages" box.
In addition to showing data as two- or three-dimensional images, the respective API libraries contain interaction (PyQtGraph, Bokeh) and data analysis (Pandas) methods. With a short sample program, I can show you in each case how to take the initial hurdles in stride. (See the "Installation and Variants" box.)
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
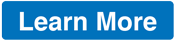
News
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.