Implementing physics in a LÖVE game
Tutorial – LÖVE Physics
Video game animation is not simply a matter of making your characters move – you also have to consider the physics of the world in which they move.
In issue 234 of Linux Magazine [1], I introduced LÖVE [2], the Lua-based framework used for creating 2D games, by drawing a character, Cubey McCubeFace, who could walk across the screen. Now I'm going to explore another aspect of LÖVE by going back into an animated world and causing an object to fall out of the sky.
As long as your game characters are moving from side to side, things are more or less easy. The moment you need them to jump or fall, things get more complicated – that is, if you have to program a physics engine yourself. Luckily, LÖVE provides a way to simulate 2D rigid bodies in a realistic manner through its physics module. In this tutorial, I'll explore how that works [3].
Landscaping
First of all you need a playing field in which things can move around and collide with each other. I'll set up a "landscape" like the one you can see in Figure 1 and by drawing the outline of the terrain first (see Listing 1).
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
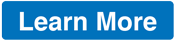
News
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.