Working with Git branches
Command Line – Git Branches
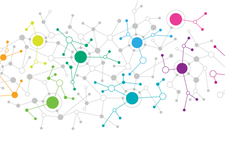
© Lead Image © Rachael Arnott, 123RF.com
Git makes version control as simple as possible. To manage your Git branches successfully, you need to learn the ins and outs of git branch and git merge.
In a previous issue of Linux Magazine [1], I outlined how to set up a Git Repository (Table 1). Once a repo is set up, you are ready to use Git [2] for version control.
Version control in Git is about managing and creating branches (collections of files that are variations of each other). By making a branch, you can edit files without altering the original. Later, if you choose, you can merge branches to create a more advanced copy.
The process of managing branches is so simple that branches tend to proliferate in projects that use Git, with branches being created for different users, subsystems or hardware architectures, each release, experiments, or any other criteria that may useful. In fact, while other version control systems also use branches, using Git repos is so flexible that it is widely considered Git's defining feature. Two Git sub-commands are the main tools for managing branches: git branch
[3], which sets up and edits branches, and git merge
[4], which combines branches.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
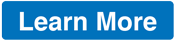
News
-
System76 Releases COSMIC Alpha 7
With scores of bug fixes and a really cool workspaces feature, COSMIC is looking to soon migrate from alpha to beta.
-
OpenMandriva Lx 6.0 Available for Installation
The latest release of OpenMandriva has arrived with a new kernel, an updated Plasma desktop, and a server edition.
-
TrueNAS 25.04 Arrives with Thousands of Changes
One of the most popular Linux-based NAS solutions has rolled out the latest edition, based on Ubuntu 25.04.
-
Fedora 42 Available with Two New Spins
The latest release from the Fedora Project includes the usual updates, a new kernel, an official KDE Plasma spin, and a new System76 spin.
-
So Long, ArcoLinux
The ArcoLinux distribution is the latest Linux distribution to shut down.
-
What Open Source Pros Look for in a Job Role
Learn what professionals in technical and non-technical roles say is most important when seeking a new position.
-
Asahi Linux Runs into Issues with M4 Support
Due to Apple Silicon changes, the Asahi Linux project is at odds with adding support for the M4 chips.
-
Plasma 6.3.4 Now Available
Although not a major release, Plasma 6.3.4 does fix some bugs and offer a subtle change for the Plasma sidebar.
-
Linux Kernel 6.15 First Release Candidate Now Available
Linux Torvalds has announced that the release candidate for the final release of the Linux 6.15 series is now available.
-
Akamai Will Host kernel.org
The organization dedicated to cloud-based solutions has agreed to host kernel.org to deliver long-term stability for the development team.