Using clean code principles for better code
Clean code is a set of rules and procedures that make it easier to read and understand source code regardless of the programming language used. Many clean code strategies fit all languages, but some more far-reaching strategies only prove useful in the object-oriented world. The clean code concept is not new or revolutionary. Back in 2008, Robert C. Martin described the procedures in his book, Clean Code: A Handbook of Agile Software Craftsmanship [1].
With today's highly complex IT applications, ensuring that source code has a clean structure has becoming increasingly important. The clean code concept has evolved in recent years, and the procedures described in Martin's book have been expanded. To get you started writing cleaner code, I'll describe some of the core ideas and rules of clean code.
The Scout's Rule
"Always leave code cleaner than you found it." If you take this rule to heart, a project's source code will get better and better over time. You don't need to be afraid of breaking something, because the previous version can always be restored thanks to the version management (i.e., Git). Modern IDEs also provide many tools for reengineering code. Renaming classes or methods doesn't pose so much of a risk anymore.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
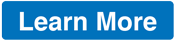
News
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.
-
KaOS 2025.05 Officially Qt5 Free
If you're a fan of independent Linux distributions, the team behind KaOS is proud to announce the latest iteration that includes kernel 6.14 and KDE's Plasma 6.3.5.