A Bash web server
One Liners
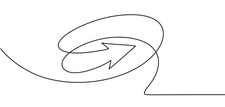
© Lead Image © valenty, 123RF.com
With one line of Bash code, you can create a Bash web server for quickly viewing the output from Bash scripts and commands.
For people who do a lot of work with command-line tools or Bash code, having a Bash web server could be very handy. I was really amazed that in one line of Bash code I was able to create web servers that could:
- Send the output from a Bash command directly to a browser page
- Create diagnostic pages using standard Linux tools
- Create pages that view Raspberry PI GPIO pins
- Create a page to toggle a Rasp PI GPIO pin
One-Line Web Servers
While a number of minimal, one-line web servers exist in most programming languages [1], you can create a Bash web server using the networking utility nc
(or netcat
) as follows:
while true; do { \ echo -ne "HTTP/1.0 200 OK\r\n \ Content-Length: \ $(wc -c <index.htm)\r\n\r\n"; \ cat index.htm; } | nc -l -p 8080 ; \ done
This Bash statement echoes a string with an HTTP header, the file content length, and an HTML file to a listener connecting on port 8080 using the cat
command to show the HTML file. This one-line Bash example shows a single page (index.htm
), which isn't overly useful. There are other web server options that would work much better.
Where a Bash web server really stands out is in its ability to execute command-line utilities or scripts and send the results to a web client.
Bash Web Server Calling Bash Commands
The Bash command output can be included in the echo
string along with the HTTP header when a client listener connects. For example, the iostat
command, which is used for system monitoring, can be viewed on a web page with:
while true; do echo \ -e "HTTP/1.1 200 OK\n\n$(iostat)" \ | nc -l -k -p 8080 -q 1; done
With this Bash statement, there are two important options that need to be set on nc
: -k
, which keeps the connection open after the first connection, and -q 1
, which closes the connection after one second, so another connection can occur. Depending on the complexity of the script that is being run, the -q
timing may need to be adjusted.
Figure 1 shows a web page with the iostat
output.
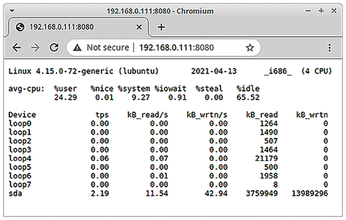
Multiple Commands with Headings
Comments and multiple command-line utilities can be combined as a variable string that can be passed to the Bash web server.
The FIGlet [2] utility is useful for custom-sized ASCII headings, which can come in handy if you don't want use HTML syntax. To install FIGlet in Ubuntu enter:
sudo apt-get install figlet
To run FIGlet, simply add the text string and use the -f
option for a font presentation:
$ figlet "123 Test" -f smslant ______ ____ ______ __ < /_ ||_ / /_ __/__ ___ / /_ / / __/_/_ < / / / -_|_-</ __/ /_/____/____/ /_/ \__/___/\__/
To get the output shown in Figure 2, Listing 1 uses FIGlet headings with the sensors
and vmstat
utilities.
Listing 1
Using FIGlet Headings
title1=$(figlet Sensors -f big) cmd1=$(sensors) title2=$(figlet VMStat -f small) cmd2=$(vmstat) thebody="$title1\n$cmd1\n$title2\n$cmd2" while true; do echo \ -e "HTTP/1.1 200 OK\n\n$thebody" \ | nc -l -p 8080 -q 1; done
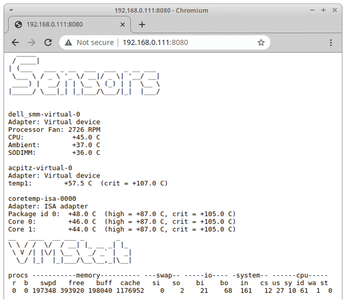
Buy this article as PDF
(incl. VAT)