Picking locks with local file inclusion
Local Job
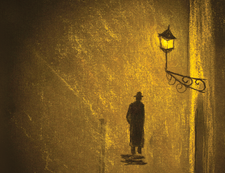
A local file inclusion attack uses files that are already on the target system.
When trying to break into a web server, ethical hackers often alter some of the variables that are present in a website's URLs. This type of attack can fall into a number of different categories. Some attacks concern the manipulation of files that a server has access to. The definition of directory traversal, as it suggests, is allowing an attacker to traverse a filesystem and then read files (that they shouldn't have access to).
On the other hand, Local File Inclusion (LFI) and Remote File Inclusion (RFI) attacks can also execute the files that they have access to. As you would guess, LFI is concerned with files that are already present on the target system (which is usually a server), whereas RFI is where an attacker uploads a malicious file (or references external files via a URL).
This article looks at my favorite way to take advantage of local file inclusion. Although this attack is not an advanced attack, when I saw how creative it was, it really opened my eyes to the ingenious methods used by attackers. This attack is a perfectly balanced combination of simplicity and guile. I also offer additional ways of delivering payloads to exploit LFI vulnerabilities and include lots of references. I'll use PHP for this article. However, the principles also apply to other server-side languages.
[...]
Buy this article as PDF
(incl. VAT)