Using a RESTful API to get data from a music database
Catching up on REST
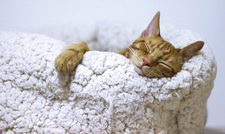
© Photo by Aleksandar Cvetanovic on unsplash
Craft the perfect URL to access metadata in an online music database.
Many network-based web services offer APIs that comply with the REpresentational State Transfer (REST) design style. APIs that comply with REST constraints are called RESTful.
RESTful APIs are attractive because they leverage existing HTTP infrastructure, the same infrastructure and protocols that browsers use. As a result, a RESTful API has access to a great variety of resources (applications, libraries, developers, etc.). From an end-user perspective, you can use your existing browser as a client to access server databases. Developers like RESTful APIs because it is easy to integrate server data and resources into applications.
Music services often use RESTful APIs to provide metadata about artists and musical tracks. Offering this data through a REST interface makes it very easy to build a client application that will receive and display the information. This article shows how to query music data through a RESTful API.
[...]
Buy this article as PDF
(incl. VAT)