Programming in Go
All Systems Go
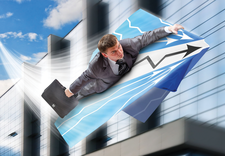
© Lead Image © nomadsoul1, 123RF.com
The Go programming language combines type safety with manageable syntax and an extensive library. We take you through a programming example.
The Go programming language recently celebrated its fourth birthday and increasing popularity. The language has very few limits when it comes to coding Unix daemons, networking code, parallelized programs, and the like, although it is probably less well-suited for an operating system kernel. The Docker container virtualization project and Ubuntu Juju tool are two examples of projects written in Go.
Designed as an heir to the C programming language [1], Go offers many of its predecessor's strengths, while simplifying syntax and supporting secure programming (e.g., through strong type casting). The Unsafe module makes Go resemble C more closely, although it does compromise security, as the module name suggests.
The standard Go library [2] is extensive, offering many useful system programming modules for data compression, cryptography, binary file formats (ELF, Mach-O), and so forth. In this article, I will create a simple tool written in Go that has a function similar to the ps
process status tool in Linux.
Go Projects
Go projects have a somewhat peculiar directory structure. To compile source code files, you must follow a certain procedure, so that the go
build and project tool work properly.
The GOPATH
environment variable specifies the directory in which all Go projects reside. Below that are dist
, bin
, and src
, the latter of which contains the source code under a directory that uniquely identifies a package or project. In principle, this can be any string but is typically your domain name, followed by a project name (e.g., linux-magazine.com/<project>
; Figure 1). The go
tool also loads projects off the Internet (e.g., from GitHub), which then end up in $GOPATH/src/github.com/<project>
.
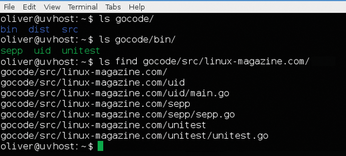
In my example, the projects reside in $HOME/gocode
. The environment variable is set like this:
export GOPATH=$HOME/gocode
The following call sets the project directory for the tool I will be programming, lap
(i.e., list all processes):
mkdir -p src/linux-magazine.com/lap
If you now store a small "Hello World" file here [1], you can compile the project as follows:
go build linux-magazine.com/lap
If you take a look at the GOPATH/bin
directory, you will discover no files there. The go
tool only copies the binary to this location if you use the install
command; it thus makes sense to do this straight away, instead of detouring via build
. Program libraries end up in GOPATH/pkg
. The object files can be removed by issuing go clean package
; with an additional switch, go clean-f
also removes the binaries.
The idea behind lap
is quite simple. In the /proc
filesystem on Linux, a globally visible directory for each running process uses the process ID as the name. Below this are a number of virtual files with information about this process, including the stat
and status
files, which contain, for example, the parent process ID, the owner, the start time, and so on.
Processing Files
The first task is therefore to list the files in /proc
and filter out the ones with the process information. It can be done quite easily with the filepath.Glob
function, which returns all the file names that match a certain pattern in an array. The following approach takes a little detour to demonstrate a few aspects of loops and string processing in Go:
entries, err := ioutil.ReadDir(procDir) for index, proc := range entries { // do something with proc }
The directory contents are returned by a call to the ReadDir
function from the ioutil
package. To go through all the entries, use the for
loop with a range expression that lets you iterate over arrays, slices, strings, maps, and channels. With only one control variable, range
assigns this to the array index. Go automatically assigns a second variable to the content of the corresponding array element.
The Go compiler only accepts this if you actually do something with the index
variable. If you don't, you can use the Go wildcard for variables, _
. A proc
in this example is of the os.FileInfo
type; it implements an interface that includes the call to Name()
for reading the file name.
Unicode Support
Finding out whether the first character is a number is a little more difficult because Go uses UTF-8 for character encoding – which is actually a good thing. Because UTF-8 is a format that uses between 1 and 4 bytes for a character, it is not clear from the outset how many bytes the first character includes. Typically, Go programmers work their way through a byte array bit by bit and then make decisions on when a new UTF-8 character starts. With a type cast, Go does this automatically in one fell swoop. This results in an array of "runes" – as single UTF-8 characters are called in Go – of which you read the first character with an array index. This in turn can be done with a simple call to the unicode.IsDigit()
function, which expects a rune as an argument:
if unicode.IsDigit([]rune(proc.Name())[0]) {...
Incidentally, the small example program that can be seen in Figure 2 proves that this is not just true of Arabic numerals in the Unicode world but also works for any other numeric systems.
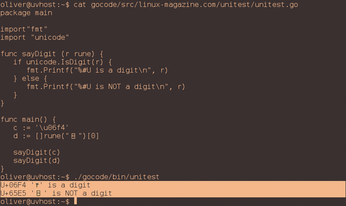
The next task is to open the virtual files in procfs and process their content. Conveniently, the ioutil
library again offers a function (ReadFile
) that fully reads a file and stores the contents in a byte array:
stat, err := ioutil.ReadFile(filename)
As you can see, the function returns two values: the content of the file and an error code. This is typical of Go functions and certainly more structured than, for example, returning a null pointer for the content in the case of an error. If you assign the error code to a variable, you also need to process the variable; otherwise, the compiler will in turn generate an error message and refuse to compile the file. If you want to ignore the return value, you can again assign the error code to the wildcard, _
. To process the fault, you would typically test whether the err
variable is equal to nil
. If so, no error has occurred.
The /proc/PID/stat
file contains only one line in a fixed format, with the individual fields separated by spaces. Unfortunately, the format is not documented anywhere in a useful way, not even in the Linux kernel documentation of procfs [2].
Ultimately, your only resource, if you want to know exactly what is going on, is to take a look at the Linux source code. The PID comes first no matter what (and this is the same as the directory name), then in brackets you have the process name, the process status, and the parent PID.
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
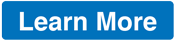
News
-
Canonical Releases Ubuntu 24.04
After a brief pause because of the XZ vulnerability, Ubuntu 24.04 is now available for install.
-
Linux Servers Targeted by Akira Ransomware
A group of bad actors who have already extorted $42 million have their sights set on the Linux platform.
-
TUXEDO Computers Unveils Linux Laptop Featuring AMD Ryzen CPU
This latest release is the first laptop to include the new CPU from Ryzen and Linux preinstalled.
-
XZ Gets the All-Clear
The back door xz vulnerability has been officially reverted for Fedora 40 and versions 38 and 39 were never affected.
-
Canonical Collaborates with Qualcomm on New Venture
This new joint effort is geared toward bringing Ubuntu and Ubuntu Core to Qualcomm-powered devices.
-
Kodi 21.0 Open-Source Entertainment Hub Released
After a year of development, the award-winning Kodi cross-platform, media center software is now available with many new additions and improvements.
-
Linux Usage Increases in Two Key Areas
If market share is your thing, you'll be happy to know that Linux is on the rise in two areas that, if they keep climbing, could have serious meaning for Linux's future.
-
Vulnerability Discovered in xz Libraries
An urgent alert for Fedora 40 has been posted and users should pay attention.
-
Canonical Bumps LTS Support to 12 years
If you're worried that your Ubuntu LTS release won't be supported long enough to last, Canonical has a surprise for you in the form of 12 years of security coverage.
-
Fedora 40 Beta Released Soon
With the official release of Fedora 40 coming in April, it's almost time to download the beta and see what's new.