Wireless control over a long distance with the LoRa modem
Tell LoRa I love her… – Ricky Valence
Some variants of the Raspberry Pi come equipped with WiFi, which make them suitable for wireless data gathering and control applications in certain circumstances. However, the range of WiFi is limited, and it generally requires a WiFi-enabled router issuing IP addresses. Beyond WiFi, a number of standards exist for long range wireless communications in the 868MHz and 915MHz license-free bands that are more suitable for embedded applications. One of these license-free bands is LoRa [1], which is widely used and supported by several silicon implementations. The silicon itself is usually packaged onto modules, which are available for sale in the $20 region. These modules are often further mounted on standard PCBs and offered as development kits by the silicon manufacturers and others. This article describes how to build LoRa into a Raspberry-Pi-based telemetry system and outlines the design of a low-cost HAT for the Pi with an integrated PCB antenna (which you can build for less than $10).
Introducing LoRa
The name LoRa is derived from "Long Range," and long range is indeed one of the system's defining characteristics. One group of users claims to have achieved a 476-mile range with a transmit power of just 25mW [2]. LoRa is also low power. In the license-free ISM bands where LoRa operates [3] [4], maximum power is limited by law. But low power is often also mandated by the need for battery operation or dependence on solar energy at a remote location. So it is this combination of low power and long range that makes LoRa attractive for telemetry applications. Extreme range figures like 476 miles should be examined with a critical eye. These results are typically achieved at high altitudes with line of sight between transmitter and receiver and other optimum conditions. However, in an urban environment, ranges of up to a mile are possible with low cost, compact hardware.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
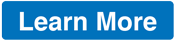
News
-
IBM Announces Powerhouse Linux Server
IBM has unleashed a seriously powerful Linux server with the LinuxONE Emperor 5.
-
Plasma Ends LTS Releases
The KDE Plasma development team is doing away with the LTS releases for a good reason.
-
Arch Linux Available for Windows Subsystem for Linux
If you've ever wanted to use a rolling release distribution with WSL, now's your chance.
-
System76 Releases COSMIC Alpha 7
With scores of bug fixes and a really cool workspaces feature, COSMIC is looking to soon migrate from alpha to beta.
-
OpenMandriva Lx 6.0 Available for Installation
The latest release of OpenMandriva has arrived with a new kernel, an updated Plasma desktop, and a server edition.
-
TrueNAS 25.04 Arrives with Thousands of Changes
One of the most popular Linux-based NAS solutions has rolled out the latest edition, based on Ubuntu 25.04.
-
Fedora 42 Available with Two New Spins
The latest release from the Fedora Project includes the usual updates, a new kernel, an official KDE Plasma spin, and a new System76 spin.
-
So Long, ArcoLinux
The ArcoLinux distribution is the latest Linux distribution to shut down.
-
What Open Source Pros Look for in a Job Role
Learn what professionals in technical and non-technical roles say is most important when seeking a new position.
-
Asahi Linux Runs into Issues with M4 Support
Due to Apple Silicon changes, the Asahi Linux project is at odds with adding support for the M4 chips.