Write your own extensions for Inkscape
Tutorial – Inkscape Scripts
Inkscape's extensions add many useful features. Here's how to write your own.
Badly documented software is common. In fact, I'd say that for free software it is almost the norm. It is also what … er … "inspires" most of my Linux Magazine articles. I set out to do something, hit the wall of insufficient or non-existent documentation, doggedly try to do it anyway, and if I succeed, hey presto, an article.
Which brings me to Inkscape's extensions. Inkscape is a wonderful piece of software, a testament to how good free and open source, community-built applications can be. However, when I began exploring it, I found the documentation of the extension engine to be staggeringly bad. For starters, a link to a Python Effect Tutorial in the Inkscape wiki leads to an empty page ("[extensions under review]") that was last "modified" in 2008!
To add insult to injury, Inkscape was recently updated to version 1.0, after being in the 0.9x circle of hell for years. Much rejoicing was to be had. Alas, with the overhaul of looks and features came an overhaul of the scripting API, and you probably guessed what happened with the docs – nothing. The little documentation there is on the API is still for the old version and is completely obsolete.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
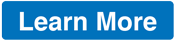
News
-
Linux Hits an Important Milestone
If you pay attention to the news in the Linux-sphere, you've probably heard that the open source operating system recently crashed through a ceiling no one thought possible.
-
Plasma Bigscreen Returns
A developer discovered that the Plasma Bigscreen feature had been sitting untouched, so he decided to do something about it.
-
CachyOS Now Lets Users Choose Their Shell
Imagine getting the opportunity to select which shell you want during the installation of your favorite Linux distribution. That's now a thing.
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.