Overview of the Serial Communication Protocol
Testing the Code
With the Arduino's serial monitor, you can enter data directly to your Arduino. After your sketch is running, open the Serial Monitor by clicking its icon. Be sure to select 9600 baud in the lower right corner (Figure 3); then, you can type L (uppercase) to turn the LED on and l (lowercase) to turn it off.
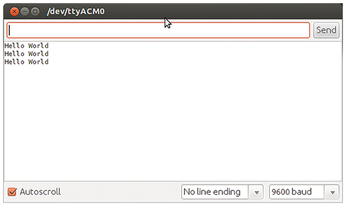
To move the servo, enter a number between 0 and 180 followed by a period. Because the Arduino doesn't know how many digits are in your number, the period lets it know that you're done sending. Sending a number and waiting five seconds also works, because Serial.setTimeout
(Listing 1, line 13) makes sure the program doesn't stall.
Independent of the output, if the button or switch changes state, you'll get a message in the serial window. If a program were to receive this serial output, it could interpret the messages and take action on the basis of the values, as shown in the Python snippet in Listing 2.
Listing 2
Python Serial Transmitter
01 import serial 02 import time 03 ser = serial.Serial('/dev/ttyAMA0') # open the Arduino serial port 04 05 while 1: 06 ser.write('L') # turn the LED on 07 time.sleep ( 1 ) # wait a second 08 ser.write ( 'l' ) # turn the LED off 09 time.sleep (1) 10 ser.close() # close port
Controlling the LED
As with any Python program, import
brings in an external library. In this case, line 1 is the interface to serial ports [4], and line 2 is the time module, which provides the sleep
function.
The serial.Serial
function opens a serial port. The only required argument is the port name. In this case, /dev/ttyAMA0
is the Arduino. Without any other parameters, communication will default to 9600 baud, 8N1 (data, parity, stop bits).
The infinite loop (lines 5-9) sends an uppercase L out the serial port (line 6), waits one second (line 7), sends a lowercase l out the serial port (line 8), and waits one second (line 9). Although line 10 will never be reached in this example, ser.close
shuts down a port. Python also takes care of the port if the program terminates.
Once you've programmed the Arduino, run the Python snippet, and your LED should start blinking. Of course, you can now change the timing or send other commands by modifying the desktop program instead of reprogramming the Arduino. This arrangement can be very handy if the Arduino is embedded in another piece of equipment (or suspended 30 feet in the air).
Listening to Inputs
The Python code in Listing 3 reports the changing states of the button and switch. The infinite loop that starts in line 4 gets a line from the serial port with ser.readline
(line 5) and splits it at the colon (line 6). To the left of the colon, the string will either be Switch
or Button
. If it is Switch
(line 7) and the status (right of the colon, statusParts[1]
) is
, then the switch is ON (line 8). Otherwise the switch is off (line 9). Lines 10-12 work the same way, except it checks and reports the state of the button.
Listing 3
Python Serial Receiver
01 import serial 02 ser = serial.Serial('/dev/ttyAMA0') # open the Arduino serial port 03 04 While 1: 05 status = ser.readline() 06 statusParts = status.split ( ":" ) 07 If statusParts [ 0 ] == "Switch": 08 If statusParts [ 1 ] == "0": print ( "The switch is ON" ) 09 Elif statusParts [ 1 ] == "1": print ( "The switch is OFF" ) 10 Elif statusParts [ 0 ] == "Button": 11 If statusParts [ 1 ] == "0": print ( "The button is pressed" ) 12 Elif statusParts [ 1 ] == "1": print ( "The button is released" )
When you run the snippet in Listing 3 and fiddle with the button and switch, you should see messages announcing the changes in state in the terminal. Although this example just shows a message, it is not hard to see how this could trigger other code.
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
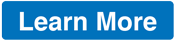
News
-
NVIDIA Released Driver for Upcoming NVIDIA 560 GPU for Linux
Not only has NVIDIA released the driver for its upcoming CPU series, it's the first release that defaults to using open-source GPU kernel modules.
-
OpenMandriva Lx 24.07 Released
If you’re into rolling release Linux distributions, OpenMandriva ROME has a new snapshot with a new kernel.
-
Kernel 6.10 Available for General Usage
Linus Torvalds has released the 6.10 kernel and it includes significant performance increases for Intel Core hybrid systems and more.
-
TUXEDO Computers Releases InfinityBook Pro 14 Gen9 Laptop
Sporting either AMD or Intel CPUs, the TUXEDO InfinityBook Pro 14 is an extremely compact, lightweight, sturdy powerhouse.
-
Google Extends Support for Linux Kernels Used for Android
Because the LTS Linux kernel releases are so important to Android, Google has decided to extend the support period beyond that offered by the kernel development team.
-
Linux Mint 22 Stable Delayed
If you're anxious about getting your hands on the stable release of Linux Mint 22, it looks as if you're going to have to wait a bit longer.
-
Nitrux 3.5.1 Available for Install
The latest version of the immutable, systemd-free distribution includes an updated kernel and NVIDIA driver.
-
Debian 12.6 Released with Plenty of Bug Fixes and Updates
The sixth update to Debian "Bookworm" is all about security mitigations and making adjustments for some "serious problems."
-
Canonical Offers 12-Year LTS for Open Source Docker Images
Canonical is expanding its LTS offering to reach beyond the DEB packages with a new distro-less Docker image.
-
Plasma Desktop 6.1 Released with Several Enhancements
If you're a fan of Plasma Desktop, you should be excited about this new point release.