Extract and analyze GPS data with Go
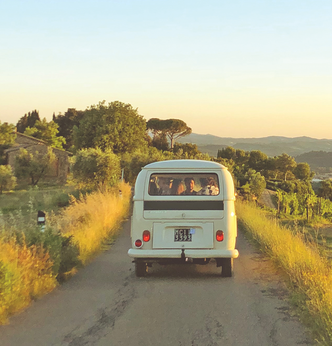
The GPX data of my hiking trails, which I recorded with the help of geotrackers and apps like Komoot [1], hold some potential for statistical analysis. On which days was I on the move, and when was I lazy? Which regions were my favorites for hiking, and in which regions on the world map did I cover the most miles?
No matter where the GPX files come from – whether recorded by a Garmin tracker or by an app like Komoot that lets you download the data from its website [2] – the recorded data just screams to be put through more or less intelligent analysis programs. For each hike or bike ride, the tours/
directory (Figure 1) contains one file in XML format (Figure 2). Each of these GPX files consists of a series of geodata recorded with timestamps. In each case, the data shows the longitude and latitude determined using GPS, from which, in turn, you can determine a point on the Earth's surface, visited at a given time.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
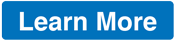
News
-
IBM Announces Powerhouse Linux Server
IBM has unleashed a seriously powerful Linux server with the LinuxONE Emperor 5.
-
Plasma Ends LTS Releases
The KDE Plasma development team is doing away with the LTS releases for a good reason.
-
Arch Linux Available for Windows Subsystem for Linux
If you've ever wanted to use a rolling release distribution with WSL, now's your chance.
-
System76 Releases COSMIC Alpha 7
With scores of bug fixes and a really cool workspaces feature, COSMIC is looking to soon migrate from alpha to beta.
-
OpenMandriva Lx 6.0 Available for Installation
The latest release of OpenMandriva has arrived with a new kernel, an updated Plasma desktop, and a server edition.
-
TrueNAS 25.04 Arrives with Thousands of Changes
One of the most popular Linux-based NAS solutions has rolled out the latest edition, based on Ubuntu 25.04.
-
Fedora 42 Available with Two New Spins
The latest release from the Fedora Project includes the usual updates, a new kernel, an official KDE Plasma spin, and a new System76 spin.
-
So Long, ArcoLinux
The ArcoLinux distribution is the latest Linux distribution to shut down.
-
What Open Source Pros Look for in a Job Role
Learn what professionals in technical and non-technical roles say is most important when seeking a new position.
-
Asahi Linux Runs into Issues with M4 Support
Due to Apple Silicon changes, the Asahi Linux project is at odds with adding support for the M4 chips.