A plotting library
Matplotlib [1] is an open source Python library for high-quality data visualization, which you can use interactively or inside scripts. While Matplotlib might look like just a supercharged version of the venerable plotting utility Gnuplot [2], that's not the whole story. Matplotlib really shines in the integration of charting with heavy numeric processing. When combined with other Python libraries, such as NumPy and pandas (see the "Matplotlib's Partners: NumPy and pandas" box), Matplotlib is often considered an effective open source alternative to MATLAB [3].
Some tools have steeper learning curves than others. In my opinion, Matplotlib's learning curve is wider, not steeper than average. For example, Matplotlib has an entire tutorial devoted to colors specifications! In other words, Matplotlib has so many features and options that it looks much more complex than it actually is. For this reason, much of this tutorial uses simplified versions of some official examples to introduce the main Matplotlib concepts and features and connects the dots between them.
Install Procedure
You will find several ways to install the most recent stable version of Matplotlib, all properly documented on its website. However, unless you really need the LATEST stable version, I strongly suggest that you save time and frustration by installing whatever binary packages are available in your preferred distribution's official repository. On Ubuntu 21.04, for example, you can install a recent Matplotlib and all its dependencies by just searching for it in the Ubuntu Software Center or, as I did, by typing this command at the prompt:
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
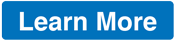
News
-
Plasma Ends LTS Releases
The KDE Plasma development team is doing away with the LTS releases for a good reason.
-
Arch Linux Available for Windows Subsystem for Linux
If you've ever wanted to use a rolling release distribution with WSL, now's your chance.
-
System76 Releases COSMIC Alpha 7
With scores of bug fixes and a really cool workspaces feature, COSMIC is looking to soon migrate from alpha to beta.
-
OpenMandriva Lx 6.0 Available for Installation
The latest release of OpenMandriva has arrived with a new kernel, an updated Plasma desktop, and a server edition.
-
TrueNAS 25.04 Arrives with Thousands of Changes
One of the most popular Linux-based NAS solutions has rolled out the latest edition, based on Ubuntu 25.04.
-
Fedora 42 Available with Two New Spins
The latest release from the Fedora Project includes the usual updates, a new kernel, an official KDE Plasma spin, and a new System76 spin.
-
So Long, ArcoLinux
The ArcoLinux distribution is the latest Linux distribution to shut down.
-
What Open Source Pros Look for in a Job Role
Learn what professionals in technical and non-technical roles say is most important when seeking a new position.
-
Asahi Linux Runs into Issues with M4 Support
Due to Apple Silicon changes, the Asahi Linux project is at odds with adding support for the M4 chips.
-
Plasma 6.3.4 Now Available
Although not a major release, Plasma 6.3.4 does fix some bugs and offer a subtle change for the Plasma sidebar.