Introducing the Zing zero-packet network utility
Zing Functionality
As Table 2 indicates, the actual functionality of the Zing network utility is contained within the method doZingToHost()
, which takes the host parameter and a port parameter to operate upon. The internal implementation uses the host and port multiple times in nested loops. The return parameter of the method doZingToHost()
is a double value (the time in milliseconds as a real number).
The method source code for doZingToHost()
is shown in Listing 2. (Note that this code is useful as a static library function.)
Listing 2
doZingToHost
01 public static double doZingToHost(final String host, final int port) { 02 03 if(inet_addr == null) { 04 inet_addr = getHostAddrName(host); 05 }//end if 06 07 try { 08 if(inet_addr.isReachable(timeout)){ //command-line option -timeout 09 System.out.printf(".. Error: Host is unreachable.%n", 10 host, inet_addr.getHostAddress()); 11 System.exit(1); 12 }//end if 13 } catch(Exception _ignore){ 14 System.out.printf(".. Error: Host contact timeout.%n", 15 host, inet_addr.getHostAddress()); 16 System.exit(1); 17 }//end try 18 19 boolean presentFlag = true; //host at socket is present, default is true 20 long socketTimeStart = 0, socketTimeClose = 0, socketTimeTotal = 0; 21 22 try { 23 socketTimeStart = System.currentTimeMillis(); 24 Socket socket = new Socket(host, port); 25 socket.setSoTimeout(timeout); 26 socket.close(); 27 socketTimeClose = System.currentTimeMillis(); 28 } catch (SocketTimeoutException _ignore){ 29 System.out.printf("Timed out after %d ms waiting for host.%n", timeout); 30 presentFlag = false; 31 } catch (Exception _ignore) { 32 presentFlag = false; 33 }//end try 34 35 if (presentFlag){ 36 socketTimeTotal = socketTimeTotal + (socketTimeClose - socketTimeStart); 37 } else { 38 System.out.print("."); 39 return -1.0d; 40 }//end if 41 42 return (double) socketTimeTotal; 43 44 }// end doZingToHost
The doZingToHost()
logic is fairly simple: Get the host name and address, and check if the host is present, unless there's an error. Zing the host by connecting and disconnecting to the host, while getting the starting and closing time to connect. If the host is present, compute the time in milliseconds as a real number (double value). Return the double value, and if the host is not present, return -1 as a sentinel value.
The core method doZingToHost()
is called by the main()
method, which will then repeatedly zing the host and accumulate timing information.
Main Core Functionality
The main core functionality of the Zing network utility is simple and consists of the main()
method that invokes the doZingToHost()
within the parameters given. The main()
method, removing the parameter check and initialization, is shown in Listing 3.
Listing 3
main()
01 inet_addr = getHostAddrName(host); 02 03 System.out.printf("ZING: %s (%s): %d ports used, %d ops per cycle%n", 04 hostName, hostAddr, ports.length, (limit * ports.length)); 05 06 long timeZingStart = System.currentTimeMillis(); 07 08 for (int x = 0; x < count; x++) { 09 10 System.out.printf("#%d ", x + 1); 11 double totalTime = 0.0; 12 System.out.print("."); 13 14 for (int y = 0; y < limit; y++) { 15 for (int port : ports) { 16 totalTime += doZingToHost(host, port); 17 } // end for(port) 18 } // end for(limit) 19 20 System.out.print("."); 21 double time = getTotalTime(totalTime, ports.length, limit); 22 System.out.print("."); 23 report(time); // time = -1.0d, absent, else active 24 } // end for(count) 25 26 long timeZingClose = System.currentTimeMillis(); 27 28 //get min, max, avg 29 double min = Double.MAX_VALUE, max = Double.MIN_VALUE, avg = 0.0; 30 for (int x = 0; x < count; x++) { 31 if (min > zingTimeTable[x]) { 32 min = zingTimeTable[x]; 33 }//end if 34 if (max < zingTimeTable[x]) { 35 max = zingTimeTable[x]; 36 }//end if 37 avg += zingTimeTable[x]; 38 }//end for 39 40 avg = avg / (double) count; 41 42 double std_dev = stddev(avg, zingTimeTable); 43 44 System.out.printf("%n--- zing summary for %s/%s ---%n", hostName, hostAddr); 45 System.out.printf("%d total ops used; total time: %d ms%n", 46 (ports.length * limit * count), (timeZingClose - timeZingStart)); 47 System.out.printf("total-time min/avg/max/stddev = %.3f/%.3f/%.3f/%.3f ms", 48 min, avg, max, std_dev); 49 System.out.printf("%n%n"); 50 51 System.exit(0);
The main()
method logic is also fairly simple:
- Get the Internet address, and check if the host is available and present.
- Print command line arguments and parameters used to zing.
- Repeatedly loop and zing the host, counting and timing the responses for each port to the limit of the Zing operation.
- Calculate the average, the standard deviation, and the minimum and maximum time value to zing a host system.
- Report and print overall timing values and metrics in the classic ping style.
There is initially a check for parameters, and if the -h
parameter is passed for help to the main()
method, the usage()
method prints the command line parameter. An example of using help with the Zing network utility is:
java -jar Zing.jar -h Usage: zing -h | [-4|-6] [-c count] [-op ops] [-p ports] [-t timeout] host Example: zing -4 -c 4 -op 4 -p 80,443 -t 4000 google.com
After reporting the usage for the Zing network utility, the app then terminates. A help-usage command line parameter overrides all other parameters and operation of the Zing network utility.
Order of Operation
The order of operation for the Zing utility begins with processing the command line interface parameters. Any unrecognized or invalid parameters are indicated. If successful, Zing then gets the host as a hostname and address.
Then the method doZingToHost()
is called within a loop that iterates over the count, the ops limit, and, for each port, a triple-nested loop. The total time is then accumulated as the three nested loops iterate.
The total time is then calculated by the method getTotalTime()
, and then the report()
method is used to report or print to the console or terminal a summary of the information determined by the Zing utility.
« Previous 1 2 3 Next »
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
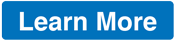
News
-
Canonical Releases Ubuntu 24.04
After a brief pause because of the XZ vulnerability, Ubuntu 24.04 is now available for install.
-
Linux Servers Targeted by Akira Ransomware
A group of bad actors who have already extorted $42 million have their sights set on the Linux platform.
-
TUXEDO Computers Unveils Linux Laptop Featuring AMD Ryzen CPU
This latest release is the first laptop to include the new CPU from Ryzen and Linux preinstalled.
-
XZ Gets the All-Clear
The back door xz vulnerability has been officially reverted for Fedora 40 and versions 38 and 39 were never affected.
-
Canonical Collaborates with Qualcomm on New Venture
This new joint effort is geared toward bringing Ubuntu and Ubuntu Core to Qualcomm-powered devices.
-
Kodi 21.0 Open-Source Entertainment Hub Released
After a year of development, the award-winning Kodi cross-platform, media center software is now available with many new additions and improvements.
-
Linux Usage Increases in Two Key Areas
If market share is your thing, you'll be happy to know that Linux is on the rise in two areas that, if they keep climbing, could have serious meaning for Linux's future.
-
Vulnerability Discovered in xz Libraries
An urgent alert for Fedora 40 has been posted and users should pay attention.
-
Canonical Bumps LTS Support to 12 years
If you're worried that your Ubuntu LTS release won't be supported long enough to last, Canonical has a surprise for you in the form of 12 years of security coverage.
-
Fedora 40 Beta Released Soon
With the official release of Fedora 40 coming in April, it's almost time to download the beta and see what's new.