Reactive Programming and the Reactive Manifesto
React
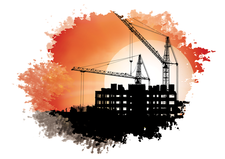
In July of last year, Jonas Bonér and some other colleagues published the Reactive Manifesto. Soon, the term was on everyone's lips, but this seemingly new programming concept is actually much older.
The term Reactive Programming was coined by David Harel and Amir Pnueli back in 1985 [1]. Harel and Pnueli focused their academic work on designing systems that must constantly respond to input or changes. Over the following years, the meaning of the term reactive programming shifted slightly. Today, an application is generally considered reactive if it responds quickly to input or data changes.
If you investigate the existing solutions that specialize in reactive programming, you will encounter a mix of terms, concepts, and implementations. Jonas Bonér and his co-authors wanted to clear up this confusion with their Reactive Manifesto [2][3]. Bonér and many of his colleagues work for Typesafe, which provides a platform for reactive programs [4], so the authors have a strong incentive to advance the prospects of reactive programming.
The Reactive Manifesto defines common terms that improve collaboration among the developer communities and also make it easier for users and manufacturers to talk to each other. According to the manifesto, a reactive program:
- Reacts to events – Even-driven nature emphasizes data flow and is easily extendable
- Reacts to load – Focuses on scalability by avoiding contention on shared resources
- Reacts to failure – Has ability to recover from an error or unexpected situation
- Reacts to users – Honors response time guarantees regardless of load
Reactive programming is especially useful with interactive programs, robotics controllers, web applications, and programs that analyze large amounts of data. Wherever a program needs to provide an answer within a few milliseconds, or must operate with high efficiency under large data volume, reactive techniques offer a compelling alternative. Because these requirements apply to more and more applications, it is not surprising that more projects are focusing on reactive programming.
Always on the Move
Programmers often need to perform a computation and store the result in a new variable. A simple example:
a=b+2
In most programming languages, the contents of the a
variable remain constant until the program explicitly takes an action to change it – even if the contents of b
change. In other words, if b
changes, a
will no longer equal b+2
unless the program repeats the operation.
In contrast, reactive programming insists on the variables a
and b
remaining dependent on each other: once b
changes, a
is automatically updated. The variable a
thus always contains the value of b+2
, regardless of the value of b
. A programmer does not have to think about where to repeat the computation. This approach reduces the sources of error and allows the application to respond faster. In this sense, you can think of the term reactive
programming as retroactive
programming.
Whereas developers in conventional languages concentrate on the control flow (i.e., the command sequence), data flow is typically in the foreground for reactive programming. The event-driven nature of reactive programming lends itself to parallel processing: The more processors an application can involve, the faster it performs its computations – at least in theory.
If the components of an application run concurrently, reactive programming makes it easier to run the program on distributed systems or in the cloud. Asynchronous data processing also helps with complex execution scenarios: After the user interface has sent the changed data to the database, the program does not wait for an answer but is immediately available for more input.
Languages
With its view toward data flow rather than commands, reactive programming is easy to integrate with existing languages. In object-oriented programming, for example, you can pass in data changes using the Observer design pattern [5]. This is the path that Microsoft takes with its Rx library, which adds reactive programming to the .NET world (Figure 1) [6].
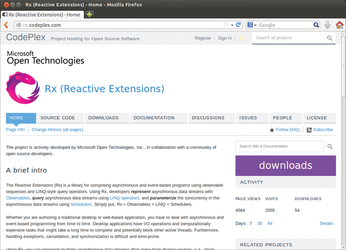
A Java port goes by the name of RxJava and comes from the online video store, Netflix [7]. For most other important languages, appropriate libraries or extensions already exist. Ruby has Frappuccino [8], and while Sodium [9] supports reactive programming in Java, Haskell and C++.
Reactive Program with the object-oriented paradigm is often referred to as Object-Oriented Reactive Programming (OORP). Reactive programming is particularly widespread in functional languages, where it is known as Functional Reactive Programming, FRP. Some languages are designed for reactive programming from the outset. A promising FRP language called Elm seeks to replace the established JavaScript language (see Figure 2 and the "Networked Elm" box).
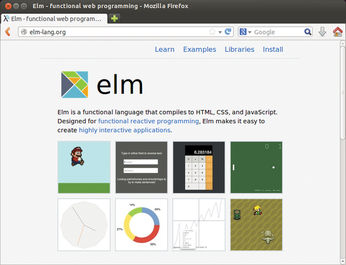
Networked Elm
The functional programming language Elm is one of the few languages whose inventors designed it from the outset for Reactive Programming. Elm focuses on programming web applications; the associated compiler creates HTML, CSS, and JavaScript [11]. The programming language was invented by Evan Czaplicki in 2011 as part of his thesis at Harvard University.
Elm does not use variables in the conventional sense. In addition to constants:
a = 2 + 4
Elm supports signals. Signals behave like variables but can suddenly change value. A prime example of this is the Mouse.position
signal, which always contains the current coordinates of the mouse pointer. If the programmer includes Mouse.position
in a computation, Elm automatically updates the result after a mouse movement. This behavior is easily illustrated by outputting the mouse position on the screen (Figure 5). The source code for outputting the mouse position contains just two lines:
import Mouse main = lift asText Mouse.position
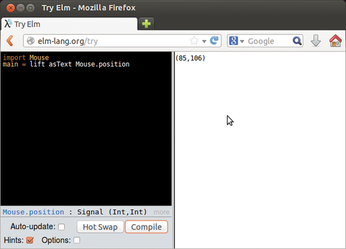
import
includes the library with the mouse functions and signals. The lift
function then dumps the current mouse coordinates from Mouse.position
into the asText
function. The asText
function, in turn, translates the numeric value into text. The text is then pushed into the special constant main
, and the contents appears on the screen. The lift
function is necessary to apply a normal function to the signal. Elm refers to this process as lifting. The result is again a signal. lift
itself assumes that the function (in this case asText
) only expects one parameter. If the function requires two parameters, the programmer must use lift2
, or lift3
for three parameters, and so on.
You can try out the code directly in the browser: The Elm developer provides a small development environment on the website [12]. You type the code on the side, and after clicking on Compile (bottom left in the window), the result – or an error message – immediately appears on the right.
Apart from its unusual lift
function, Elm is little reminiscent of Haskell or CoffeeScript. Thanks to the libraries included with Elm, you can quickly implement client applications in the browser; signals predestine Elm for building interactive user interfaces right from the outset. In contrast to many other functional programming languages, however, the transition from imperative languages is quite simple. For a quick and easy-to-understand introduction, check out the online documentation [13]. The sample collection [14] shows what is possible with Elm. For example, you can change the diagram in Figure 6 with the mouse in real time; a complete pong game requires only 100 lines of code [15]. However, Elm still down not appear in any serious practical applications.
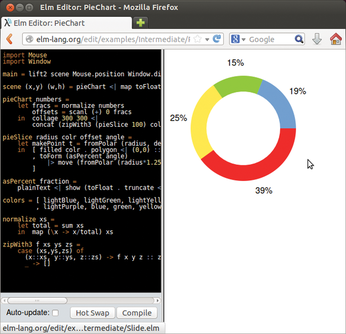
One for All
According to the manifesto, an application is considered reactive if it can respond immediately to a stimulus. To this end, all the software components must be event-driven, scalable, resilient, and responsive. In a web application, for example, not just the client browser but also the server components must respond quickly; otherwise, the entire system grinds to a halt.
The components of a reactive application can only communicate with each other via events (message passing); they must also send and receive asynchronously. This mandatory requirement ensures that the components run concurrently and thus that the application scales more easily.
Blocking operations are forbidden. If a thread grabs exclusive access to a resource, other threads have to wait, and in the worst case, this prevents any further execution of the application. To avoid such situations, the manifesto prohibits blocking operations, synchronization, and resource hogging. The intent is for the system to use this approach to achieve lower latency and higher throughput under heavy load. Any necessary synchronization should be handled by the runtime environment. Developers only have to deal with how the individual components of their applications interact.
To prevent bottlenecks occurring somewhere at high load, it is important for the complete system to work asynchronously and in a non-blocking way. In other words, not only must the GUI be able to handle asynchronously transmitted events, but also the middleware, right down to the underlying database. The authors of the manifesto call for programs to be "reactive from top to bottom." If you follow all the rules, your application can display the official "We Are Reactive" banner (Figure 3).
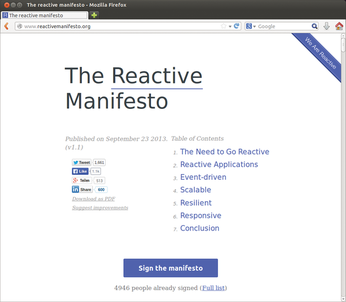
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
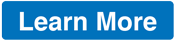
News
-
Canonical Releases Ubuntu 24.04
After a brief pause because of the XZ vulnerability, Ubuntu 24.04 is now available for install.
-
Linux Servers Targeted by Akira Ransomware
A group of bad actors who have already extorted $42 million have their sights set on the Linux platform.
-
TUXEDO Computers Unveils Linux Laptop Featuring AMD Ryzen CPU
This latest release is the first laptop to include the new CPU from Ryzen and Linux preinstalled.
-
XZ Gets the All-Clear
The back door xz vulnerability has been officially reverted for Fedora 40 and versions 38 and 39 were never affected.
-
Canonical Collaborates with Qualcomm on New Venture
This new joint effort is geared toward bringing Ubuntu and Ubuntu Core to Qualcomm-powered devices.
-
Kodi 21.0 Open-Source Entertainment Hub Released
After a year of development, the award-winning Kodi cross-platform, media center software is now available with many new additions and improvements.
-
Linux Usage Increases in Two Key Areas
If market share is your thing, you'll be happy to know that Linux is on the rise in two areas that, if they keep climbing, could have serious meaning for Linux's future.
-
Vulnerability Discovered in xz Libraries
An urgent alert for Fedora 40 has been posted and users should pay attention.
-
Canonical Bumps LTS Support to 12 years
If you're worried that your Ubuntu LTS release won't be supported long enough to last, Canonical has a surprise for you in the form of 12 years of security coverage.
-
Fedora 40 Beta Released Soon
With the official release of Fedora 40 coming in April, it's almost time to download the beta and see what's new.