Zack's Kernel News
Zack's Kernel News
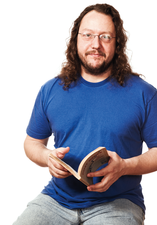
Chronicler Zack Brown reports on the latest news, views, dilemmas, and developments within the Linux kernel community.
Fixing Memory Usage by Not Fixing It
Al Viro recently posted some "flagday" patches – changes that were so invasive, they couldn't be done piecemeal. His idea was to convert the kernel memory handling APIs so that functions like free_page()
and a bunch of others would return a pointer instead of just a plain number. His thinking was that everyone doing anything with RAM wanted to get a usable memory pointer, instead of having to do a typecast every time they called the memory handling functions.
Linus Torvalds, however, put the kibosh on the whole idea. Changing an API that had been in place for almost the entire lifespan of the Linux kernel project would cause a lot of confusion among developers. It would also, he said, make backporting new features to earlier versions of the kernel an even bigger headache than it already was, because the backport would have to make sure it undid all of Al's flagday changes, just to get a patch that would successfully apply to the earlier kernel version.
The proper way to do what Al had proposed, said Linus, would be to create a new set of functions that had different names and to allow both versions of each function to exist side by side. That way, people could migrate their portions of the kernel to the new functions in a piecemeal way over time.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
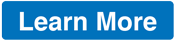
News
-
Linux Hits an Important Milestone
If you pay attention to the news in the Linux-sphere, you've probably heard that the open source operating system recently crashed through a ceiling no one thought possible.
-
Plasma Bigscreen Returns
A developer discovered that the Plasma Bigscreen feature had been sitting untouched, so he decided to do something about it.
-
CachyOS Now Lets Users Choose Their Shell
Imagine getting the opportunity to select which shell you want during the installation of your favorite Linux distribution. That's now a thing.
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.