A Go password manager for the terminal
Programming Snapshot – Go Password Manager
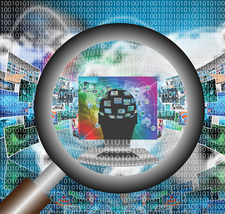
© Lead Image © Tatiana Venkova, 123RF.com
A Go application for the terminal helps Mike Schilli remember his passwords.
Whether it's on a Post-it note under the screen or in a commercial application like OnePass, users have to write down their passwords somewhere. The Go application for the terminal presented in this article encrypts the sensitive data for storage on the hard drive and displays selected entries after entering the master password. The secret data leaves traces only in the computer's memory, with the traces vanishing when the program is closed.
Resourceful users could simply put all account names and passwords in a text file and encrypt it. However, to add new entries, the file would have to be decrypted and then re-encrypted after editing. To ensure that no unencrypted data remains on the drive, you would then need to run a scrub command to overwrite the deleted file after every edit. After decryption, all of the passwords would come up at the same time, emblazoned on the screen, where a passing colleague with sharp eyesight might catch a glimpse of one or more of them.
A number of password apps manage passwords in an exemplary manner, but do you really want to trust a random company with your confidential data and rely on them not to make mistakes during encryption or data management? Let's not forget that apps like OnePass hit you with significant monthly fees, and a guy like me has to count my pennies. The passview program (pv
) presented in this article manages an encrypted collection of passwords and displays a selected entry in a terminal user interface (UI) after entering the master password (Figure 1). You can scroll through the entries and pick out the one you want before its sensitive data actually appears at the push of a button.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
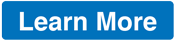
News
-
Linux Hits an Important Milestone
If you pay attention to the news in the Linux-sphere, you've probably heard that the open source operating system recently crashed through a ceiling no one thought possible.
-
Plasma Bigscreen Returns
A developer discovered that the Plasma Bigscreen feature had been sitting untouched, so he decided to do something about it.
-
CachyOS Now Lets Users Choose Their Shell
Imagine getting the opportunity to select which shell you want during the installation of your favorite Linux distribution. That's now a thing.
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.