Web programming with ECMAScript 6
New Script
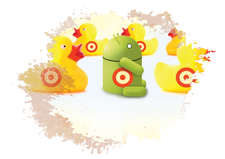
The new ECMAScript 6 language eliminates many historical problems associated with JavaScript.
In 1995, Netscape and Sun Microsystems announced the official arrival of the JavaScript scripting language. According to the original press release, JavaScript was intended as "… an open, cross-platform object scripting language for the creation and customization of applications on enterprise networks and the Internet." [1] The goal was to create a scripting language for the emerging HTML-based Internet that would run easily in the browser client context and would also have uses on the server side. The subsequent browser wars led to many battles over the role of JavaScript as a core technology for the Internet. Microsoft developed its own JavaScript-like language, which they called JScript, and the two implementations bore a striking resemblance but were just different enough to cause problems for web developers trying to write cross-platform programs.
The ECMA-262 specification emerged as an attempt to standardize JavaScript-like languages, so programmers would be able to operate independently of a single vendor. The programming language standardized in the ECMA-262 specification came to be known as ECMAScript [2]. ECMAScript still exists as a universal JavaScript-like language tailored for web development environments. The ECMAScript project website even calls ECMAScript "the language of the web," [3] and the standard is still an important means for understanding and predicting the evolution of web technologies.
JavaScript, JScript, and other alternatives such as Adobe's ActionScript, all offer compatibility with ECMA-262 and the universal ECMAScript language. The current version of the language is ECMAScript version 5, but version 6 is already available in draft form, and support for ECMAScript 6 is starting to appear in many popular browsers. Version 6 actually addresses some problems associated with contemporary versions of JavaScript
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
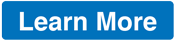
News
-
System76 Releases COSMIC Alpha 7
With scores of bug fixes and a really cool workspaces feature, COSMIC is looking to soon migrate from alpha to beta.
-
OpenMandriva Lx 6.0 Available for Installation
The latest release of OpenMandriva has arrived with a new kernel, an updated Plasma desktop, and a server edition.
-
TrueNAS 25.04 Arrives with Thousands of Changes
One of the most popular Linux-based NAS solutions has rolled out the latest edition, based on Ubuntu 25.04.
-
Fedora 42 Available with Two New Spins
The latest release from the Fedora Project includes the usual updates, a new kernel, an official KDE Plasma spin, and a new System76 spin.
-
So Long, ArcoLinux
The ArcoLinux distribution is the latest Linux distribution to shut down.
-
What Open Source Pros Look for in a Job Role
Learn what professionals in technical and non-technical roles say is most important when seeking a new position.
-
Asahi Linux Runs into Issues with M4 Support
Due to Apple Silicon changes, the Asahi Linux project is at odds with adding support for the M4 chips.
-
Plasma 6.3.4 Now Available
Although not a major release, Plasma 6.3.4 does fix some bugs and offer a subtle change for the Plasma sidebar.
-
Linux Kernel 6.15 First Release Candidate Now Available
Linux Torvalds has announced that the release candidate for the final release of the Linux 6.15 series is now available.
-
Akamai Will Host kernel.org
The organization dedicated to cloud-based solutions has agreed to host kernel.org to deliver long-term stability for the development team.