Discover how to use and probe a SQLite database
SQLite [1] (pronounced sequel-lite or S-Q-L-lite) is a public domain, embedded, relational database engine that runs on everything from smartphones to mainframes. If you use Linux or any other modern operating system, chances are good that you are already using at least one SQLite database. That alone is reason enough to learn the basics of SQLite, and it is in your interest to know not just how to back up that data, but how to generate, process, and analyze it in ways that would not be possible with other applications.
Moreover, you can install SQLite with almost zero configuration or manual work and then run it without root privileges. Finally, despite its simplicity, SQLite can handle even huge quantities of data, which means it may even help you on the job someday.
What You Will Learn
In this tutorial, I explain what SQLite is, discuss how it works, and look at a few practical ways in which to use it. Although I show only a few quick examples of actual database queries, you can find plenty of those at the SQLite website or from online cheat sheets. Instead, I focus on basic SQLite management, concepts, components, where you can find SQLite data on your desktop or smartphone, and why you might want to process it. Basic knowledge of the command line and shell scripts is all you need to take advantage of this tutorial.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
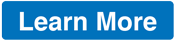
News
-
Plasma Ends LTS Releases
The KDE Plasma development team is doing away with the LTS releases for a good reason.
-
Arch Linux Available for Windows Subsystem for Linux
If you've ever wanted to use a rolling release distribution with WSL, now's your chance.
-
System76 Releases COSMIC Alpha 7
With scores of bug fixes and a really cool workspaces feature, COSMIC is looking to soon migrate from alpha to beta.
-
OpenMandriva Lx 6.0 Available for Installation
The latest release of OpenMandriva has arrived with a new kernel, an updated Plasma desktop, and a server edition.
-
TrueNAS 25.04 Arrives with Thousands of Changes
One of the most popular Linux-based NAS solutions has rolled out the latest edition, based on Ubuntu 25.04.
-
Fedora 42 Available with Two New Spins
The latest release from the Fedora Project includes the usual updates, a new kernel, an official KDE Plasma spin, and a new System76 spin.
-
So Long, ArcoLinux
The ArcoLinux distribution is the latest Linux distribution to shut down.
-
What Open Source Pros Look for in a Job Role
Learn what professionals in technical and non-technical roles say is most important when seeking a new position.
-
Asahi Linux Runs into Issues with M4 Support
Due to Apple Silicon changes, the Asahi Linux project is at odds with adding support for the M4 chips.
-
Plasma 6.3.4 Now Available
Although not a major release, Plasma 6.3.4 does fix some bugs and offer a subtle change for the Plasma sidebar.