Interactive Scripts
Core Technology
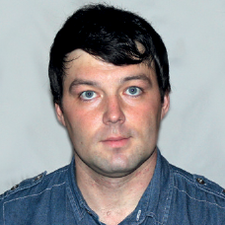
Some shell scripts are silent; others communicate to users extensively. Learn how to make their dialog smoother with, er … , dialogs.
As an administrator, you may view shell scripts as wordless minions that do their job and die silently – unless an error occurs, of course. At least, that's what we expect from a well-behaving Unix command. There are good reasons for that (think pipelining), but it doesn't mean you must have it that way all the time.
In this Core Tech, we'll learn some tricks to make shell scripts interact with the user via command prompts and dialog boxes. This is how you got Slackware installed, right? (You did try Slackware, didn't you?) Yes, the Slackware installer is basically a shell script, and with some tools under your belt, you can do no worse than Patrick Volkerding. Sound good? If so, let's go and have some text-mode fun.
Command Prompt
Perhaps the simplest form of interactivity you can have in your scripts is a command prompt. That's how shell itself is made interactive, after all. Getting pieces of data from the user is possible with the read
built-in command [1]. But, to make things fancier, let's throw some history support and other readline goodies into the mix.
[...]
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
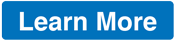
News
-
Linux Hits an Important Milestone
If you pay attention to the news in the Linux-sphere, you've probably heard that the open source operating system recently crashed through a ceiling no one thought possible.
-
Plasma Bigscreen Returns
A developer discovered that the Plasma Bigscreen feature had been sitting untouched, so he decided to do something about it.
-
CachyOS Now Lets Users Choose Their Shell
Imagine getting the opportunity to select which shell you want during the installation of your favorite Linux distribution. That's now a thing.
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.