Using OpenSCAD to build custom 3D pieces Build Your Own Body
Tutorials – OpenSCAD
OpenSCAD lets you use simple scripts to build 3D bodies from primitive shapes that you can then send to your 3D printer. It also lets you create custom shapes for pieces and objects. In this article, we look at two ways to do just that.
In last month's installment [1], you saw how to use primitive 3D bodies (cubes, spheres, cylinders, etc.) to build complex 3D objects. Apart from combining basic primitives, there are more ways of making bodies in OpenSCAD [2]. One is to extrude them from 2D shapes; another is to build them from a bunch of vertices and faces you define in arrays.
Let's see how both of these work by building an "arm" that will let you attach a sports camera to a printer's hotbed. This arm could look something like what you can see in Figure 1. With the arm attached to the bed, you will be able to monitor your print with the action always in the center of the frame.
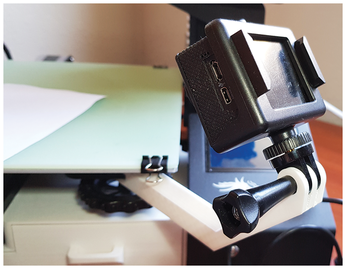
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
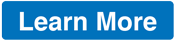
News
-
Linux Hits an Important Milestone
If you pay attention to the news in the Linux-sphere, you've probably heard that the open source operating system recently crashed through a ceiling no one thought possible.
-
Plasma Bigscreen Returns
A developer discovered that the Plasma Bigscreen feature had been sitting untouched, so he decided to do something about it.
-
CachyOS Now Lets Users Choose Their Shell
Imagine getting the opportunity to select which shell you want during the installation of your favorite Linux distribution. That's now a thing.
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.