A digital picture frame with weather forecast
Reuse and Recycle
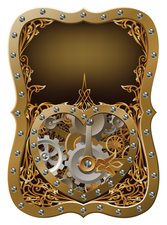
© Lead Image © Christos Georghiou, 123RF.com
A digital picture frame displays photographs and a current weather forecast with just a few hundred lines of Bash and a Raspberry Pi.
Not all that long ago I was thinking it would be pretty neat to buy the small-form-factor SheevaPlug computer [1]. However, it was pretty pricey for a computer for which I had no specific use, so I never bought it. All of that went out the window when the pretty powerful and super affordable Raspberry Pi came out in 2012. Although I didn't have any immediate project in mind, I lined up with everyone else to purchase one.
Once I received my Raspberry Pi, I set out to create projects (e.g., a proxy server, LED cube [2], and networked music device [3]) just to get started. I also experimented with the I2C and SPI protocols and played with devices previously in the domain of the Arduino.
Before I knew it, I had a small collection of these neat little devices. Over time, however, they fell out of use. These classic Raspberry Pis still work, but they aren't as fast and as capable as the most recent Raspberry Pi. While visiting my parents last summer, I saw their old unused TFT monitor in the corner, and it occurred to me how it could be reused. A 15-inch monitor is nothing special when connected to a PC, but it is pretty fabulous when turned into an electronic picture frame.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
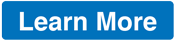
News
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.
-
KaOS 2025.05 Officially Qt5 Free
If you're a fan of independent Linux distributions, the team behind KaOS is proud to announce the latest iteration that includes kernel 6.14 and KDE's Plasma 6.3.5.
-
Linux Kernel 6.15 Now Available
The latest Linux kernel is now available with several new features/improvements and the usual bug fixes.
-
Microsoft Makes Surprising WSL Announcement
In a move that might surprise some users, Microsoft has made Windows Subsystem for Linux open source.
-
Red Hat Releases RHEL 10 Early
Red Hat quietly rolled out the official release of RHEL 10.0 a bit early.
-
openSUSE Joins End of 10
openSUSE has decided to not only join the End of 10 movement but it also will no longer support the Deepin Desktop Environment.
-
New Version of Flatpak Released
Flatpak 1.16.1 is now available as the latest, stable version with various improvements.