Create a custom Raspberry Pi OS image
To get a Raspberry Pi up and running, you need to download the latest operating system (OS) release image, burn it to an SD card, slide the card into the Pi, and power it up. After the Pi boots, you log in with the default credentials and run sudo raspi-config
to configure WiFi, locale, keyboard, and time zone and to enable SSH, I2C, a camera, and whatever else you need for I/O. Next, you update the OS with sudo apt update
, sudo apt upgrade
, and reboot before finally logging back in and installing all your favorite software that is not installed on the base image.
For casual Pi users, this procedure is a one-time or rare task. However, for experienced makers who have gone through this drill dozens – if not hundreds – of times, it is a real pain.
In this article, I present a method to create your own custom Raspberry Pi OS image that is just under 2GB in size, making it very fast to burn to any size SD card. No more waiting for an 8 or 16GB image to burn. This custom image not only burns fast, but when it boots, it expands the root filesystem to the full size of the target SD card. When the boot completes, you have a clean Pi OS system with your preferred customizations. No more raspi-config
and wasting time installing your preferred software.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
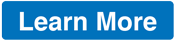
News
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.