A plotting library
Data Visualization
Matplotlib offers so many options that you may have trouble deciding on which ones to use for your plots.
Matplotlib [1] is an open source Python library for high-quality data visualization, which you can use interactively or inside scripts. While Matplotlib might look like just a supercharged version of the venerable plotting utility Gnuplot [2], that's not the whole story. Matplotlib really shines in the integration of charting with heavy numeric processing. When combined with other Python libraries, such as NumPy and pandas (see the "Matplotlib's Partners: NumPy and pandas" box), Matplotlib is often considered an effective open source alternative to MATLAB [3].
NumPy and pandas
NumPy [4] is the core library for scientific computing in Python. As far as Matplotlib is concerned, NumPy's most important components are the high-performance data structures used in some examples of this tutorial and the functions to manage them. Pandas [5] is another library, specializing in manipulation of numerical tables and time series. The combination of Matplotlib, NumPy, and pandas for numeric processing is really powerful, but it is also a relatively advanced topic that would be impossible to cover properly in just one tutorial for beginners. However, Matplotlib is really useful even alone. What you will learn with this tutorial will make it easier to add NumPy and pandas later on.
Some tools have steeper learning curves than others. In my opinion, Matplotlib's learning curve is wider, not steeper than average. For example, Matplotlib has an entire tutorial devoted to colors specifications! In other words, Matplotlib has so many features and options that it looks much more complex than it actually is. For this reason, much of this tutorial uses simplified versions of some official examples to introduce the main Matplotlib concepts and features and connects the dots between them.
Install Procedure
You will find several ways to install the most recent stable version of Matplotlib, all properly documented on its website. However, unless you really need the LATEST stable version, I strongly suggest that you save time and frustration by installing whatever binary packages are available in your preferred distribution's official repository. On Ubuntu 21.04, for example, you can install a recent Matplotlib and all its dependencies by just searching for it in the Ubuntu Software Center or, as I did, by typing this command at the prompt:
#> sudo apt-get install python3-Matplotlib
Coding Styles
There are two distinct coding styles for writing and (re)using Matplotlib code: object-oriented and pyplot.
The first style (Listing 1) uses classical object-oriented programming in any language: First you create objects (fig
and ax
, whose meaning I will explain shortly), and then you invoke "methods" associated to them (e.g., ax.plot
) to do the actual plotting.
Listing 1
Object-Oriented Style
fig, ax = plt.subplots(figsize=(5, 2.7)) ax.plot(x, x, label='linear') # Create a plot ax.legend(); # Add a legend.
The second style (Listing 2) uses pyplot functions (in which the plt
prefix means "use this function provided by the pyplot module") to create and manage charts similar to MATLAB. Listing 2, in fact, does exactly the same things as Listing 1 but by calling stateful functions such as figure
, plot
, or legend
(instead of methods attached to an instance of some object). In this context, "stateful" means that each pyplot function call adds or changes something to the current state of a plot, without erasing what has been done by previous calls of other functions.
Listing 2
Pyplot Style
plt.figure(figsize=(5, 2.7)) plt.plot(x, x, label='linear') plt.legend();
In practice, pyplot function calls have equivalent Matplotlib object-oriented methods, and vice versa, to such an extent that your choice of coding style is a matter of personal preference. However, the official Matplotlib documentation suggests using the pyplot style for "quick interactive work" and using the object-oriented style, which is more flexible, for code that will be reused often in many different ways.
Matplotlib Terminology
Before looking at any actual code, I need to explain some basic terminology, because Matplotlib gives a few words a slightly different meaning than they have in ordinary English.
Aside from what you see on the screen, Matplotlib uses "Figure" as a keyword that creates a container of objects called "Artists."
Basically, everything visible inside a Matplotlib Figure is an Artist, from drawings to embedded images, text captions, and so on. Each Figure keeps track of, and connects, all its child Artists, including nested subfigures, non-graphic objects such as titles or legends, and, of course, "Axes."
An Axes (plural!) is maybe the most basic and most essential Matplotlib Artist: It is a region, directly attached to a specific Figure, where you can plot some dataset. You can create Axes together with their parent Figure or add them later.
Each Axes has at least a title and two labels, one per axis. Most other Artists cannot be moved to, or shared with, an Axes (that is, one specific plot or subplot) different from the one they were originally attached to.
Another essential Matplotlib term, "Axis" (singular), has pretty much the same meaning it has in normal English. An Axis object sets all the components of one axis of a plot, from the scale (linear, logarithmic, or other) to the extreme points, marks (also called ticks), and their corresponding text labels. Each Axes includes at least two Axis objects, but it is possible to add more, as shown later in this tutorial.
From figure borders to colors, there are a zillion ways to style each single detail of any Matplotlib plot and two high-level ways to set them. The quick and dirty option, which may be enough in some scenarios, is to manually set all the options you need when you generate a plot. The other option is to use full styles, which are predefined listings of all the default visual settings that your plots will need. You may load either one of the predefined styles shipped with Matplotlib using the style.use
function:
plt.style.use('someMatplotlibStyle')
You can also load a custom style sheet from a file that you have created. In both cases, changing styles will only require you to change the one line of code that contains the style name or the path to the file that stores it. In addition, because both methods will ignore any non-visual setting that may be inside a style, styles are portable across machines with very different installations of Matplotlib.
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
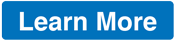
News
-
NVIDIA Released Driver for Upcoming NVIDIA 560 GPU for Linux
Not only has NVIDIA released the driver for its upcoming CPU series, it's the first release that defaults to using open-source GPU kernel modules.
-
OpenMandriva Lx 24.07 Released
If you’re into rolling release Linux distributions, OpenMandriva ROME has a new snapshot with a new kernel.
-
Kernel 6.10 Available for General Usage
Linus Torvalds has released the 6.10 kernel and it includes significant performance increases for Intel Core hybrid systems and more.
-
TUXEDO Computers Releases InfinityBook Pro 14 Gen9 Laptop
Sporting either AMD or Intel CPUs, the TUXEDO InfinityBook Pro 14 is an extremely compact, lightweight, sturdy powerhouse.
-
Google Extends Support for Linux Kernels Used for Android
Because the LTS Linux kernel releases are so important to Android, Google has decided to extend the support period beyond that offered by the kernel development team.
-
Linux Mint 22 Stable Delayed
If you're anxious about getting your hands on the stable release of Linux Mint 22, it looks as if you're going to have to wait a bit longer.
-
Nitrux 3.5.1 Available for Install
The latest version of the immutable, systemd-free distribution includes an updated kernel and NVIDIA driver.
-
Debian 12.6 Released with Plenty of Bug Fixes and Updates
The sixth update to Debian "Bookworm" is all about security mitigations and making adjustments for some "serious problems."
-
Canonical Offers 12-Year LTS for Open Source Docker Images
Canonical is expanding its LTS offering to reach beyond the DEB packages with a new distro-less Docker image.
-
Plasma Desktop 6.1 Released with Several Enhancements
If you're a fan of Plasma Desktop, you should be excited about this new point release.