RFID reader on a Raspberry Pi
Setting Up the Display
The sample program Display.py
shown in Listing 4 is designed to display the text passed in as a parameter on the display. The system controls the screen with the help of the ST7735 library [5], which was specially developed to transfer image data to the display.
Listing 4
Display.py
01 from PIL import Image 02 from PIL import ImageDraw 03 from PIL import ImageFont 04 import ST7735 05 import sys 06 07 display = ST7735.ST7735(port=1, cs=0, dc=23, backlight=None, rst=16, width=128, height=160, rotation=0, invert=False, offset_left=0, offset_top=0 ) 08 image = Image.new('RGB', (display.width, display.height)) 09 draw = ImageDraw.Draw(image) 10 print (len(sys.argv)) 11 if len(sys.argv) < 2: 12 print ("Usage: Display.py <TEXT>") 13 sys.exit() 14 draw.text((0, 70), sys.argv[1], font=ImageFont.truetype("UbuntuMono-RI",10), fill=(255, 255, 255)) 15 display.display(image)
To generate the appropriate image data, access the Pillow [6] image processing library. Pillow (also known as PIL) is a very powerful library with a large number of functions, of which I will only be using a few. The documentation [7] will give you a rough idea of what you can do with Pillow.
When launched, the Display.py
script first imports (lines 1 through 5) the ST7735 library, the required parts of Pillow, and the sys library, which lets you read the arguments from the command line. After that, the code creates a display
object with parameters suitable for the hardware you are using (line 7).
Line 8 generates an image of a size suitable for the display and stores it in the image
object. The commands that follow generate text from the parameter passed in at the command line and insert the text into the image (line 14). The last command then transfers the image to the display.
If you run the program with the
python3 Display.py "Hello world!"
command, the Hello world! output immediately pops up on the screen.
Configuring the RFID Reader
The program in Listing 5 reads the individual UIDs from the RFID chips (line 10) and displays them on the screen (line 13). The display is addressed by an external call to avoid the libraries for the display and the reader getting in each other's way. After outputting the UID, the program reads the first four data blocks from the RFID chip in a loop (starting in line 14) and displays them, too.
Listing 5
RFID Reader
01 import os 02 from pirc522 import RFID 03 rdr = RFID() 04 05 while True: 06 rdr.wait_for_tag() 07 (error, tag_type) = rdr.request() 08 if not error: 09 print("Tag detected") 10 (error, uid) = rdr.anticoll() 11 if not error: 12 print("UID: " + str(uid)) 13 os.system('python Display.py "'+str(uid)+' "') 14 for block in [0,4,8,12]: 15 (error,data)=rdr.read(block) 16 print("[ %02d]:"%(block),end="") 17 for c in data: 18 if c<=15: 19 print ("0%x "%(c),end="") 20 else: 21 print ("%x "%(c),end="") 22 print("|",end="") 23 for c in data: 24 if c>65 and c<123: 25 print (chr(c),end="") 26 else: 27 print(" ",end="") 28 print("|") 29 # Calls GPIO cleanup 30 rdr.cleanup()
Note that the reader does not authenticate against the chip, which means that the sample program can only read unprotected chips. If you want to delve a little deeper into the topic of RFID authentication, you can refer to the RC522 library documentation [8].
I used several smartphone apps to program the chips. NFC Tag Reader [9] is great for getting started and offers numerous functions, although it does have a lot of annoying advertising.
The ad-free team of NFC TagInfo [10] and NFC TagWriter [11] delivers a plethora of information that can be a bit confusing for newcomers; however, breaking the functions down into two apps makes the tools easier to use.
The MIFARE Classic Tool [12] is optimized for working with RFID chips from Mifare and supports authentication. The S50 card included with the reader kit I used in the test, and the RFID tag also included, both support this method.
Conclusions
This article can provide a basis for your own experiments with RFID chips. For many applications, simply reading the tags is enough to get a result. The Pillow library, which I used for the screen output, is also well worth a closer look. Its capabilities go far beyond simply popping up text on a display.
Infos
- RC522 RFID kit: https://www.amazon.com/SunFounder-Mifare-Reader-Arduino-Raspberry/dp/B07KGBJ9VG/
- ST7735 SPI TFT display: https://www.amazon.com/Display-Module-4-Wire-Driver-128x160/dp/B07QLY3ZB1/
- RFID tags: https://www.amazon.com/NTAG215-Compatible-Programmable-NFC-Enabled-Devices/dp/B08Z7P7L3R/
- Files for this project: https://linuxnewmedia.thegood.cloud/s/5Rzx9tQW2FJ6N3Z
- ST7735 library: https://github.com/pimoroni/st7735-python
- Pillow: https://python-pillow.org/
- Pillow documentation: https://pillow.readthedocs.io/en/stable/
- RFID Reader library: https://github.com/ondryaso/pi-rc522
- NFC tag reader: https://play.google.com/store/apps/details?id=com.gonext.nfcreader
- NFC TagInfo by NXP: https://play.google.com/store/apps/details?id=com.nxp.taginfolite
- NFC TagWriter by NXP: https://play.google.com/store/apps/details?id=com.nxp.nfc.tagwriter
- MIFARE Classic Tool: https://play.google.com/store/apps/details?id=de.syss.MifareClassicTool
« Previous 1 2
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
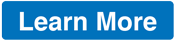
News
-
Endless OS 6 has Arrived
After more than a year since the last update, the latest release of Endless OS is now available for general usage.
-
Fedora Asahi 40 Remix Available for Macs with Apple Silicon
If you've been anticipating KDE's Plasma 6 for your Apple Silicon-powered Mac, then you're in luck.
-
Red Hat Adds New Deployment Option for Enterprise Linux Platforms
Red Hat has re-imagined enterprise Linux for an AI future with Image Mode.
-
OSJH and LPI Release 2024 Open Source Pros Job Survey Results
See what open source professionals look for in a new role.
-
Proton 9.0-1 Released to Improve Gaming with Steam
The latest release of Proton 9 adds several improvements and fixes an issue that has been problematic for Linux users.
-
So Long Neofetch and Thanks for the Info
Today is a day that every Linux user who enjoys bragging about their system(s) will mourn, as Neofetch has come to an end.
-
Ubuntu 24.04 Comes with a “Flaw"
If you're thinking you might want to upgrade from your current Ubuntu release to the latest, there's something you might want to consider before doing so.
-
Canonical Releases Ubuntu 24.04
After a brief pause because of the XZ vulnerability, Ubuntu 24.04 is now available for install.
-
Linux Servers Targeted by Akira Ransomware
A group of bad actors who have already extorted $42 million have their sights set on the Linux platform.
-
TUXEDO Computers Unveils Linux Laptop Featuring AMD Ryzen CPU
This latest release is the first laptop to include the new CPU from Ryzen and Linux preinstalled.