Keep control of goroutines with a Context construct
Programming Snapshot – Go Context
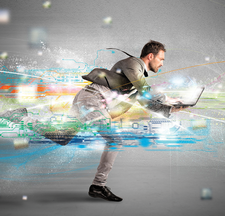
© Lead Image © alphaspirit, 123RF.com
When functions generate legions of goroutines to do subtasks, the main program needs to keep track and retain control of ongoing activity. To do this, Mike Schilli recommends using a Context construct.
Go's goroutines are so cheap that programmers like to fire them off by the dozen. But who cleans up the mess at the end of the day? Basically, Go channels lend themselves to communication. A main program may need to keep control of many goroutines running simultaneously, but a message in a channel only ever reaches one recipient. Consequently, the communication partners in this scenario rely on a special case.
If one or more recipients in Go try to read from a channel but block because there is nothing there, then the sender can notify all recipients in one fell swoop by closing the channel. This wakes up all the receivers, and their blocking read functions return with an error value.
This is precisely the procedure commonly used by the main program to stop subroutines that are still running. The main program opens a channel and passes it to each subroutine that it calls; the subroutines in turn attach blocking read functions to it. When the main program closes the channel later on, the blocks are resolved, and the subroutines proceed to do their agreed upon cleanup tasks – usually releasing all resources and exiting.
[...]
Buy this article as PDF
(incl. VAT)