Developing multimedia applications with DCCP
Over the past few years, developers have unveiled a new generation of network applications that transmit and receive multimedia content over the Internet. New multimedia applications based on technologies such as Voice over IP, Internet radio, online gaming, and video conferencing are becoming increasingly popular thanks to the availability of development libraries and the abundance of high-speed networks.
In the past, most Internet applications have used either the Transmission Control Protocol (TCP) or the User Datagram Protocol (UDP) to manage communication at the Transport layer of the TCP/IP protocol stack, but multimedia developers now have an alternative to TCP and UDP. IETF recently standardized the Datagram Congestion Control Protocol (DCCP) (RFC4340) [1], a new transport protocol designed to transmit congestion-controlled multimedia content. DCCP is becoming very popular for multimedia data transmission, mainly because it is more effective than UDP at sharing the available bandwidth.
In this article, I examine the DCCP protocol and show how to enable DCCP in Linux. Also, I will explain how to use the GStreamer DCCP plugin to create a simple client-server DCCP application.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
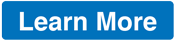
News
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.
-
KaOS 2025.05 Officially Qt5 Free
If you're a fan of independent Linux distributions, the team behind KaOS is proud to announce the latest iteration that includes kernel 6.14 and KDE's Plasma 6.3.5.