Programming with Boo
Boo Speak
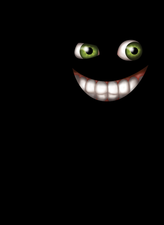
© Piumadaquila, Fotolia
Hooked on Python's "wrist-friendly" syntax? Enamored of .NET architecture but equally appreciative of C#'s strong typing? Boo offers the best of three worlds.
Unlike other development platforms, the .NET framework can mix and match code from any number of programming languages. For those who program in .NET, the universal code form known as Microsoft Intermediate Language (MSIL) is a lingua franca. If you can translate your source code into MSIL, you can combine it with, say, Visual BASIC.NET or C# to produce one executable.
Indeed, given such flexibility, developers have adapted many popular languages to .NET. IronPython [1] is a full implementation of Python for .NET, and IronRuby [2] is a proposed .NET-ready implementation of Ruby. Also, you can find Java, Lisp, and Smalltalk for .NET. Moreover, if you don't like any of the existing programming languages, you can create your own. If you can consume source code and produce MSIL, the sky is the limit.
In fact, that's the genesis of Boo [3]. Hooked on Python's sparse, "wrist-friendly" syntax but enamored of the .NET architecture and the strong typing found in C#, developer Rodrigo Barreto de Oliveira set out to combine the best features of both – with just the right amount of Ruby – into something readily suited to iterative development.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
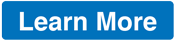
News
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.