A LÖVE animation primer
Tutorial – LÖVE animation
LÖVE is an extension of the Lua language, designed to make developing games easy. In this tutorial, we'll explore this framework by creating some animated sprites.
The topic of game design can (and does) fill entire books, so we aren't going to tackle all of that today. Instead, we'll focus on a specific project, creating animated sprites in LÖVE [1], a Lua-based [2] framework that provides you with hundreds of tools and a coherent template for creating 2D games. By creating a simple animation cycle and having LÖVE show it in a window, we will explore the fundamentals of LÖVE and help you start thinking of the games you can create with the platform.
Running LÖVE
You can download LÖVE from the project's main page or use your software manager, as it is included with most mainstream distros. The current version is LÖVE 11.3.
When you run the LÖVE interpreter for the first time from the command line with love
, you will initially see a screen that says "no game."
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
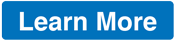
News
-
System76 Releases COSMIC Alpha 7
With scores of bug fixes and a really cool workspaces feature, COSMIC is looking to soon migrate from alpha to beta.
-
OpenMandriva Lx 6.0 Available for Installation
The latest release of OpenMandriva has arrived with a new kernel, an updated Plasma desktop, and a server edition.
-
TrueNAS 25.04 Arrives with Thousands of Changes
One of the most popular Linux-based NAS solutions has rolled out the latest edition, based on Ubuntu 25.04.
-
Fedora 42 Available with Two New Spins
The latest release from the Fedora Project includes the usual updates, a new kernel, an official KDE Plasma spin, and a new System76 spin.
-
So Long, ArcoLinux
The ArcoLinux distribution is the latest Linux distribution to shut down.
-
What Open Source Pros Look for in a Job Role
Learn what professionals in technical and non-technical roles say is most important when seeking a new position.
-
Asahi Linux Runs into Issues with M4 Support
Due to Apple Silicon changes, the Asahi Linux project is at odds with adding support for the M4 chips.
-
Plasma 6.3.4 Now Available
Although not a major release, Plasma 6.3.4 does fix some bugs and offer a subtle change for the Plasma sidebar.
-
Linux Kernel 6.15 First Release Candidate Now Available
Linux Torvalds has announced that the release candidate for the final release of the Linux 6.15 series is now available.
-
Akamai Will Host kernel.org
The organization dedicated to cloud-based solutions has agreed to host kernel.org to deliver long-term stability for the development team.