Creating your own custom maps
Maps are terribly important. Without maps we would not know where to go or, in many cases, what to do, either. Accurate maps are essential to planning anything – from a romantic weekend to large-scale urban projects. But maps are also beautiful in and of themselves. If they are customized by their owner, they can become little pieces of art. In this tutorial, I introduce a relatively simple way to generate such art using OpenStreetMap [1] and free and open source software. The results include maps in several styles and shapes that can be used as wall posters; illustrations in brochures and other documents; decorations for mugs, pillows, and other household items; or … just be used on the road, as good old paper maps!
OpenStreetMap
If you have used the Internet at all in the past 15 years, very likely you already know what OpenStreetMap is. But just in case you don't, here is a super-quick definition, focused on the aspect that is directly relevant for this tutorial: OpenStreetMap (OSM) is the Wikipedia concept applied to digital cartography, an online map of the whole world, built and continuously updated by thousands of volunteers from all around the world. It is hard to explain how important OSM is for our society, but anyone interested may find food for thought on this matter in my blog [2].
Here, what really matters is the fact that all the OSM raw data is available for reuse under an open source license and can be downloaded automatically through equally open programming interfaces. It's this availability that makes drawing custom digital maps possible, even for non-programmers and for commercial purposes.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
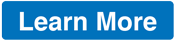
News
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.