Program a game of bingo with ReportLab and Panda3D for Python
Enable Mouse
When setting up a 3D world it is often just easier to drive the camera around and find the object you are looking for. To do so, remove the disableMouse
on line 29 so you can use the built-in camera controls. Once you have found your object, call getCam
, which uses pprint
(line 63) to output the camera position, which can then be added to the code as needed.
Tiles
The initTiles
section (lines 65-103) creates all of the 3D objects that represent bingo numbers and shuffles them to prepare for calling. To start, self.tiles
(line 66) is a list of strings that represent the tiles, and self.tiles3d
(line 67) is a dictionary of 3D objects. The key is the string from the self.tiles
list.
Bingo tiles start with one letter from the word "BINGO" and then one of 15 numbers. Column 1 (B) goes from 1 to 15, column 2 (I) from 16 to 30, and so on.
Line 69 creates bingoWord = "BINGO"
, and line 70 initializes total
to
. Line 71 loops over each character in bingoWord
and creates 15 tiles for each (line 72). The next line then creates the tile by starting with char
, the current character from bingoWord
, and appending a number created by the loop counter i
plus the accumulated total
plus 1
(otherwise it would start at 0). Once that is done, total
increments by 15 before the loop moves on to the next column.
Lines 76 and 77 are identical to the earlier code in __init__
to load a font. Although the same font loads here, it is included again so that the tiles can be a different font from the title.
Now that you have a list of tiles, they are all turned into 3D objects. Line 79 initializes oldLetter
to blank, then line 80 starts the loop over self.tiles
. Lines 81-88 create a 3D text object the same way as in __init__
, then lines 89-93 check which column a tile is in from its first letter (tile [ 0 ]
) and assigns the x
for each column.
Line 95 checks to see whether oldLetter
has changed (have you moved to the next column?), and if so, lines 96 and 97 set z=20
and update oldLetter
. Once that is done, line 99 sets the calculated x
and z
values with setPos
(line 99). The y coordinate is hard-coded at -50
because everything is the same distance from the camera. The setTwoSided
line (100) makes it so that if a letter is flipped around backward it will still look right, before decrementing z
(line 101) and adding the tile to the self.tiles3d
dictionary.
Finally, random.shuffle
on line 103 makes sure the tiles are in a random order. Now it's time to play bingo!
Play Bingo!
The callTile
section (lines 105-121) calls the tile, which will fly up close to the camera so it appears really big (Figure 4) and say the name of the tile before it flies back down to the other side of the screen to show what is now a called number (Figure 5).
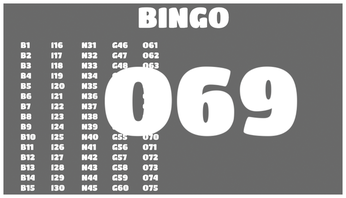
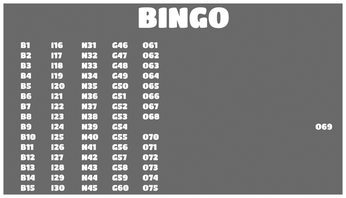
Line 106 checks self.auto
and manual
to see whether auto calling is currently active or whether this was called manually. If neither are true, then nothing should be done and the line returns. Then, len ( self.tiles) > 0
checks to make sure tiles are available. If a tile is available, it is stored in tile
; otherwise, it encounters the return
(lines 108, 109).
Now a couple of things need to be set up to move the tile around. Because this function works on all 60 tiles, the first thing you need to know is the location the tile starts, which you find out by calling getPos
on the 3D tile object stored in self.tiles3d
(line 111). The new position of the tile will be the x position plus 17,
with the same y and z coordinates calculated on line 112.
After that, the lerps (linear interpolations) need to be set up. The lerps' job is, for any slice of time, to calculate where the object is located between two positions. By default, a lerp starts with the object's current position, but you can override that if needed. The lerp also wants to know where to go and how long it takes to get there. You can optionally provide arguments to change the starting position or starting and ending behavior, or even to provide a function to calculate movement on the fly.
Line 114 is the lerp to move the bingo number up close to the camera over two seconds. Line 115 uses the newly calculated x position and flies the number from right in front of the camera down to a position on the right-hand side of the screen.
So far you have told the tile how to move, but you haven't actually moved it yet. You can think of lerps as dance moves. You can learn how to do each step, but then you have to put them together in the right order to perform the whole dance. That is where Sequence
comes in. It takes a set of lerps and executes them in the order provided, so line 117 starts with the lerp i
that moves from the initial position up to the camera. Then the special Wait
function delays for the provided number of seconds, and park
finishes in the position on the right side of the screen where you want the tile to land. If this sequence were to be used repeatedly, you could store it in a variable, but here, just call start
so the movement begins.
Line 118 calls self.speak
in a new thread, which lets the speech and the movement happen at the same time.
To wrap up, append tile
to self.calledTiles
(line 120) and then, if auto calling is on (self.auto == True
), call self.autoCall
again (line 121) to schedule callTile
to be run in five seconds.
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
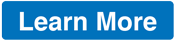
News
-
Canonical Releases Ubuntu 24.04
After a brief pause because of the XZ vulnerability, Ubuntu 24.04 is now available for install.
-
Linux Servers Targeted by Akira Ransomware
A group of bad actors who have already extorted $42 million have their sights set on the Linux platform.
-
TUXEDO Computers Unveils Linux Laptop Featuring AMD Ryzen CPU
This latest release is the first laptop to include the new CPU from Ryzen and Linux preinstalled.
-
XZ Gets the All-Clear
The back door xz vulnerability has been officially reverted for Fedora 40 and versions 38 and 39 were never affected.
-
Canonical Collaborates with Qualcomm on New Venture
This new joint effort is geared toward bringing Ubuntu and Ubuntu Core to Qualcomm-powered devices.
-
Kodi 21.0 Open-Source Entertainment Hub Released
After a year of development, the award-winning Kodi cross-platform, media center software is now available with many new additions and improvements.
-
Linux Usage Increases in Two Key Areas
If market share is your thing, you'll be happy to know that Linux is on the rise in two areas that, if they keep climbing, could have serious meaning for Linux's future.
-
Vulnerability Discovered in xz Libraries
An urgent alert for Fedora 40 has been posted and users should pay attention.
-
Canonical Bumps LTS Support to 12 years
If you're worried that your Ubuntu LTS release won't be supported long enough to last, Canonical has a surprise for you in the form of 12 years of security coverage.
-
Fedora 40 Beta Released Soon
With the official release of Fedora 40 coming in April, it's almost time to download the beta and see what's new.